Locale-Sensitive Date Formatting with SimpleDateFormat
Formatting dates in Java can be tailored to different locales to meet regional preferences. The SimpleDateFormat class provides basic formatting options, but customizing these options for specific locales poses a challenge.
Challenge:
Create a Java program that formats dates in different ways based on the locale. For example, English users should see "Nov 1, 2009" ("MMM d, yyyy"), while Norwegian users should see "1. nov. 2009" ("d. MMM. yyyy").
Proposed Solution:
The initial intention was to add format strings paired with locales to SimpleDateFormat. However, this is not feasible.
Alternative Solution:
Instead of constructing your own patterns with SimpleDateFormat, use DateFormat.getDateInstance(int style, Locale locale). This method creates a DateFormatter object preconfigured for a specific style and locale.
For example, the following code creates two DateFormatter objects, one for English and one for Norwegian:
Locale englishLocale = Locale.ENGLISH; DateFormat englishDateFormatter = DateFormat.getDateInstance(DateFormat.SHORT, englishLocale); Locale norwegianLocale = new Locale("no", "NO"); DateFormat norwegianDateFormatter = DateFormat.getDateInstance(DateFormat.SHORT, norwegianLocale);
Using these DateFormatter objects, dates can be formatted according to the specified locales:
Date date = new Date(); String englishFormattedDate = englishDateFormatter.format(date); String norwegianFormattedDate = norwegianDateFormatter.format(date);
This approach ensures that dates are formatted in a culturally appropriate manner based on the specified locales.
The above is the detailed content of How Do You Format Dates in Java for Different Locales?. For more information, please follow other related articles on the PHP Chinese website!
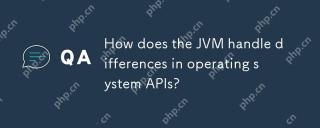
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
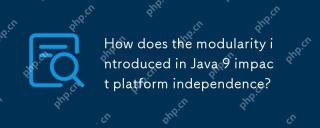
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
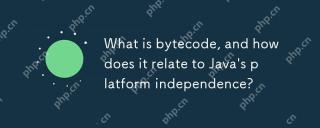
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf
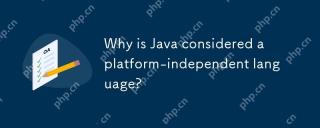
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),whichexecutesbytecodeonanydevicewithaJVM.1)Javacodeiscompiledintobytecode.2)TheJVMinterpretsandexecutesthisbytecodeintomachine-specificinstructions,allowingthesamecodetorunondifferentp
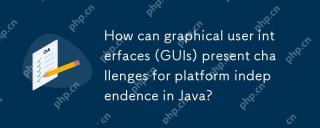
Platform independence in JavaGUI development faces challenges, but can be dealt with by using Swing, JavaFX, unifying appearance, performance optimization, third-party libraries and cross-platform testing. JavaGUI development relies on AWT and Swing, which aims to provide cross-platform consistency, but the actual effect varies from operating system to operating system. Solutions include: 1) using Swing and JavaFX as GUI toolkits; 2) Unify the appearance through UIManager.setLookAndFeel(); 3) Optimize performance to suit different platforms; 4) using third-party libraries such as ApachePivot or SWT; 5) conduct cross-platform testing to ensure consistency.
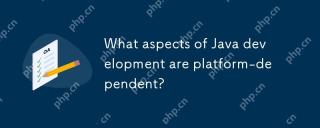
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
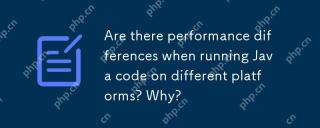
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
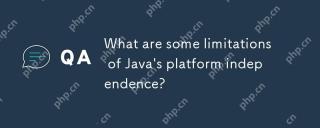
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
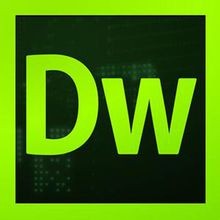
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
