


How to Securely Encrypt and Decrypt Data Using 3DES in Java
Introduction
3DES (Triple Data Encryption Standard) is a powerful encryption algorithm commonly used to safeguard sensitive data. Implementing 3DES encryption in Java is essential for various security-conscious applications. This tutorial will guide you through the steps to effectively encrypt and decrypt data using 3DES in Java.
A Common Pitfall and Solution
It's crucial to note that the code provided in the question encounters an issue where the decrypted string does not match the original string. This could result from several factors, including incorrect key handling or misconceptions about encoding and decoding.
The corrected code below addresses these issues and clarifies the usage of encoding and decoding:
import java.security.MessageDigest; import java.util.Arrays; import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; public class TripleDESTest { public static void main(String[] args) throws Exception { String text = "kyle boon"; byte[] codedtext = new TripleDESTest().encrypt(text); String decodedtext = new TripleDESTest().decrypt(codedtext); System.out.println(codedtext); // Encrypted result in byte array System.out.println(decodedtext); // Decrypted and readable string } public byte[] encrypt(String message) throws Exception { byte[] md5 = MessageDigest.getInstance("MD5").digest("HG58YZ3CR9".getBytes("utf-8")); byte[] keyBytes = Arrays.copyOf(md5, 24); for (int j = 0, k = 16; j <p><strong>Understanding the Code</strong></p><p>By creating a main method, we demonstrate how to encrypt and decrypt a sample string. The encrypt method utilizes a MD5 digest of the password "HG58YZ3CR9" to generate a 24-byte key, which is then used to initialize the DESede cipher. The encoded byte array is returned as the encrypted result.</p><p>The decrypt method performs the reverse process using the same key. By converting the decrypted byte array to a String with UTF-8 encoding, we obtain the original plaintext.</p><p><strong>Conclusion</strong></p><p>Implementing 3DES encryption in Java follows a straightforward process. By addressing potential pitfalls and understanding the underlying concepts of key handling and encoding, you can securely protect sensitive data in your applications.</p>
The above is the detailed content of How can I securely encrypt and decrypt data using 3DES in Java, and what are the common pitfalls to avoid?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
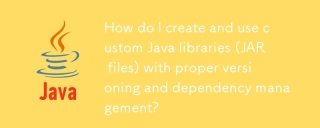
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
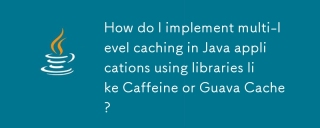
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
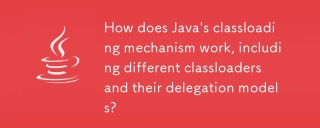
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
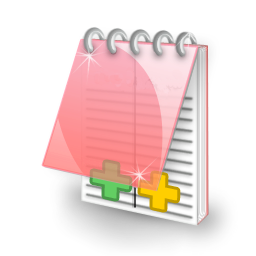
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
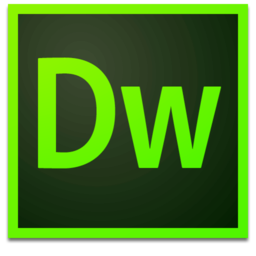
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor