


Python-Style Generators in Go
Understanding Channel Buffers
In your code, you observed that increasing the channel buffer size from 1 to 10 enhanced performance by reducing context switches. This concept is correct. A larger buffer allows the fibonacci goroutine to fill multiple spots in advance, reducing the need for constant communication between goroutines.
Channel Lifetime and Memory Management
However, a channel's lifetime is distinct from the goroutines that use it. In your original code, the fibonacci goroutine is not terminated, and the channel reference is retained in the main function. As such, the channel and its contents persist in memory, leading to a potential memory leak.
An Alternative Generator Implementation
To avoid memory leaks while still utilizing Python-style generators, you can implement a solution similar to the following:
package main import "fmt" func fib(n int) chan int { c := make(chan int) go func() { x, y := 0, 1 for i := 0; i <p><strong>Explanation:</strong></p>
- The fib function returns a channel that generates the Fibonacci sequence up to the specified n value.
- The goroutine started in the fib function constantly generates and sends Fibonacci numbers to the channel until the sequence is exhausted.
- The close(c) statement closes the channel when the sequence is complete, signaling to the main function that there are no more elements to read.
- In the main function, using a range-based for loop on the channel automatically consumes its elements until it is closed.
This approach ensures that the fibonacci goroutine terminates gracefully, preventing memory leaks and providing a clean and efficient generator implementation.
The above is the detailed content of How to Implement Python-Style Generators in Go While Avoiding Memory Leaks?. For more information, please follow other related articles on the PHP Chinese website!
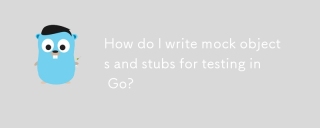
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
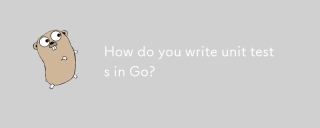
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
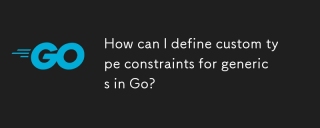
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
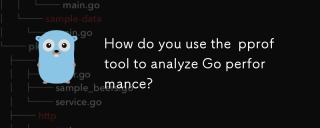
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
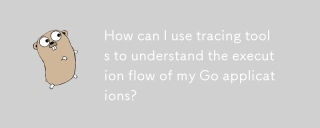
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
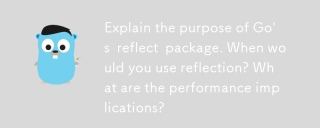
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
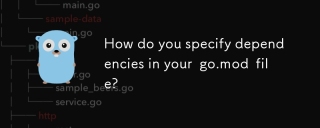
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
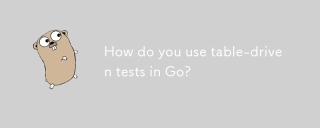
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
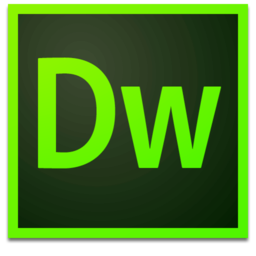
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
