Individual and Non-Continuous JTable Cell Selection
Allowing users to select multiple non-continuous cells in a JTable can be a valuable feature for various applications. Here's a detailed exploration of the approaches to achieving this functionality:
Built-in Options
The standard JTable component does not provide a direct option for selecting multiple non-continuous cells. However, there are two methods that can be leveraged in combination:
- setCellSelectionEnabled(true): This method allows individual cell selection.
- setSelectionModel(new DefaultListSelectionModel()): This method overrides the default row selection model with a list selection model, which supports non-continuous selection.
However, this approach only allows the selection of consecutive cells in a single row or column.
Modifying Mouse Event Handling
An alternative approach is to modify the JTable's mouse event handling. By overriding the processMouseEvent method, you can force the JTable to treat any mouse click with the Control key pressed as a cell selection toggle. This allows you to select individual cells regardless of their position.
import java.awt.Component; import java.awt.event.InputEvent; import java.awt.event.MouseEvent; import javax.swing.JTable; public class TableSelection extends JTable { @Override protected void processMouseEvent(MouseEvent e) { int modifiers = e.getModifiers() | InputEvent.CTRL_MASK; MouseEvent myME = new MouseEvent((Component) e.getSource(), e.getID(), e.getWhen(), modifiers, e.getX(), e.getY(), e.getXOnScreen(), e.getYOnScreen(), e.getClickCount(), e.isPopupTrigger(), e.getButton()); super.processMouseEvent(myME); } }
By using this approach, you gain the ability to select individual and non-continuous cells in your JTable. Note that this does not require any modifications to the ListSelectionModel or the JTable's selection mode.
External Selection Model
As suggested in the question, implementing your own ListSelectionModel provides complete control over the selection mechanism. This approach offers the highest flexibility and allows you to customize the selection behavior as needed.
Caveats
It's important to note that some JTable operations, such as sorting or filtering, may not work as expected with non-continuous cell selection. Additionally, you may need to handle the coloring or styling of selected cells manually.
Conclusion
While JTable does not natively support non-continuous cell selection, the aforementioned techniques provide effective solutions to achieve this functionality. By understanding the limitations and customizing the appropriate method, you can enhance your JTable's user experience and cater to specific application requirements.
The above is the detailed content of How to Enable Non-Continuous Cell Selection in a JTable?. For more information, please follow other related articles on the PHP Chinese website!
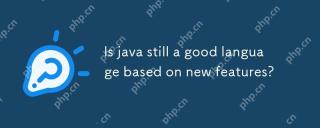
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
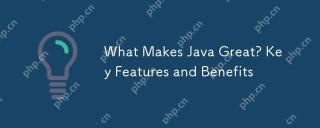
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
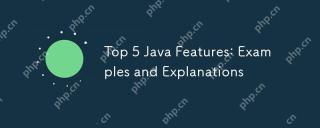
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.
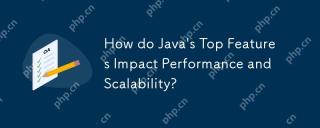
Java'stopfeaturessignificantlyenhanceitsperformanceandscalability.1)Object-orientedprincipleslikepolymorphismenableflexibleandscalablecode.2)Garbagecollectionautomatesmemorymanagementbutcancauselatencyissues.3)TheJITcompilerboostsexecutionspeedafteri
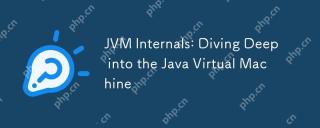
The core components of the JVM include ClassLoader, RuntimeDataArea and ExecutionEngine. 1) ClassLoader is responsible for loading, linking and initializing classes and interfaces. 2) RuntimeDataArea contains MethodArea, Heap, Stack, PCRegister and NativeMethodStacks. 3) ExecutionEngine is composed of Interpreter, JITCompiler and GarbageCollector, responsible for the execution and optimization of bytecode.
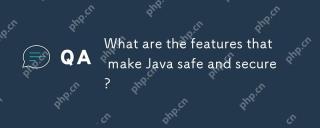
Java'ssafetyandsecurityarebolsteredby:1)strongtyping,whichpreventstype-relatederrors;2)automaticmemorymanagementviagarbagecollection,reducingmemory-relatedvulnerabilities;3)sandboxing,isolatingcodefromthesystem;and4)robustexceptionhandling,ensuringgr
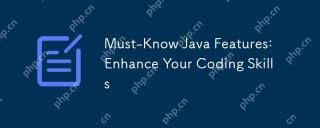
Javaoffersseveralkeyfeaturesthatenhancecodingskills:1)Object-orientedprogrammingallowsmodelingreal-worldentities,exemplifiedbypolymorphism.2)Exceptionhandlingprovidesrobusterrormanagement.3)Lambdaexpressionssimplifyoperations,improvingcodereadability
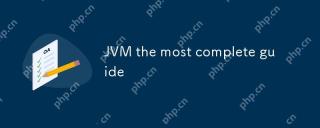
TheJVMisacrucialcomponentthatrunsJavacodebytranslatingitintomachine-specificinstructions,impactingperformance,security,andportability.1)TheClassLoaderloads,links,andinitializesclasses.2)TheExecutionEngineexecutesbytecodeintomachineinstructions.3)Memo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
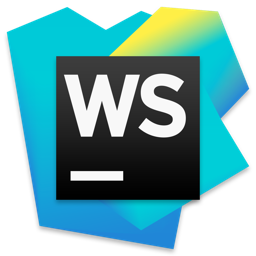
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
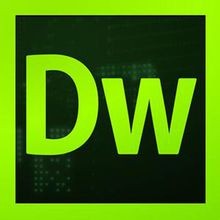
Dreamweaver CS6
Visual web development tools
