


When working with large result sets in PHP: Should I use PDO::fetchAll or PDO::fetch?
PDO::fetchAll vs. PDO::fetch: Performance Trade-Offs
When working with large result sets in PHP databases, developers may encounter the choice between using PDO::fetchAll() and PDO::fetch() in a loop. This decision primarily revolves around performance and memory considerations.
PDO::fetchAll()
- Fetches all rows from the result set in one operation.
- Efficient for extracting large datasets, minimizing database round-trips.
- However, requires significant memory to hold the entire result set.
PDO::fetch()
- Fetches individual rows in a loop.
- More memory-efficient than PDO::fetchAll().
- Suitable for cases where data is processed incrementally.
Performance Comparison
A benchmark demonstrated that PDO::fetchAll() is faster than PDO::fetch() in a loop, particularly for large result sets. However, this performance advantage comes at the cost of consuming significantly more memory.
Memory Considerations
The memory requirement of PDO::fetchAll() is proportional to the size of the result set. For large datasets, this can lead to memory exhaustion or performance issues. PDO::fetch(), on the other hand, does not require significant memory, as it processes rows sequentially.
Factors Influencing the Choice
The appropriate choice between PDO::fetchAll() and PDO::fetch() depends on:
- Size of the Result Set: If the result set is large, PDO::fetchAll() is faster but may require significant memory.
- Memory Availability: If memory is limited, PDO::fetch() in a loop is the more memory-efficient option.
- Data Processing: If data is processed incrementally, PDO::fetch() allows for more control and flexibility.
Example:
To illustrate the trade-offs, consider the following benchmark code:
$dbh = new PDO(...); $sql = 'SELECT * FROM test_table'; $stmt = $dbh->query($sql); $start_all = microtime(true); $data = $stmt->fetchAll(); $end_all = microtime(true); $start_one = microtime(true); while($data = $stmt->fetch()) {} $end_one = microtime(true); echo 'Result : ' . PHP_EOL; echo 'fetchAll : ' . ($end_all - $start_all) . 's, ' . memory_get_usage() . 'b' . PHP_EOL; echo 'fetch : ' . ($end_one - $start_one) . 's, ' . memory_get_usage() . 'b' . PHP_EOL;
The fetchAll method takes 0.35 seconds and requires 100MB of memory, while the fetch loop takes 0.39 seconds and consumes only 440 bytes of memory.
The above is the detailed content of When working with large result sets in PHP: Should I use PDO::fetchAll or PDO::fetch?. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
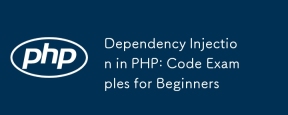
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
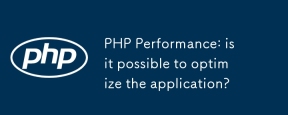
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
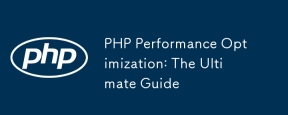
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
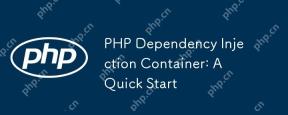
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
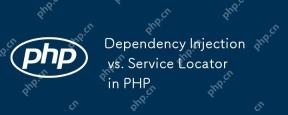
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
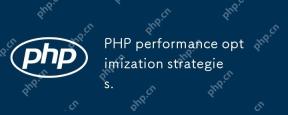
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
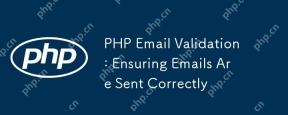
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
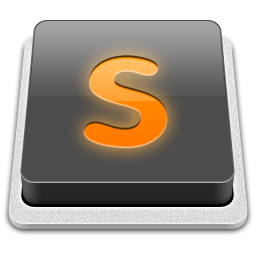
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
