Efficient Overflow Detection in Go for Integer Calculations
When working with integers, particularly in the context of a Lisp language, the concern arises about potential integer overflows that can lead to incorrect results. Detecting such overflows is crucial for maintaining the correctness of your computations.
One common approach to detecting overflows is to convert the operands to a larger integer type before performing the operation. While this guarantees overflow detection, it can be an inefficient and memory-intensive process for basic arithmetic operations.
A more efficient and precise technique for overflow detection is to utilize the mathematical properties of integer addition. For instance, when adding two 32-bit integers, an overflow occurs when the result exceeds the maximum value of a 32-bit integer (2^31-1) or falls below the minimum value (-2^31).
Consider the following code snippet to detect integer overflows in Go:
package main import ( "errors" "fmt" "math" ) var ErrOverflow = errors.New("integer overflow") func Add32(left, right int32) (int32, error) { if right > 0 { if left > math.MaxInt32-right { // Check for positive overflow return 0, ErrOverflow } } else { if left <p>In this code snippet, we define a custom function Add32 that takes two 32-bit integers as input and checks for overflow before performing the addition operation. Using mathematical principles, we determine the conditions that indicate an overflow (when the result exceeds the maximum or falls below the minimum allowed values).</p><p>If an overflow is detected, the function returns an error indicating the overflow. Otherwise, it returns the result of the addition operation.</p><p>Running this code snippet will output the following:</p><pre class="brush:php;toolbar:false">integer overflow 2147483327 2147483327 0
This indicates that an overflow occurred when adding the two 32-bit integers, and the Add32 function correctly detected and reported the error. This approach provides an efficient and reliable way to detect integer overflows in Go, ensuring the correctness of your computations.
The above is the detailed content of How to Efficiently Detect Integer Overflow in Go?. For more information, please follow other related articles on the PHP Chinese website!
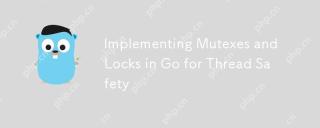
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
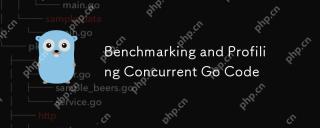
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
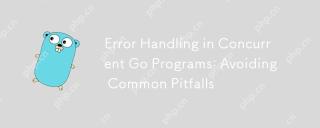
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
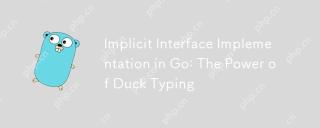
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
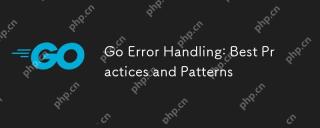
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
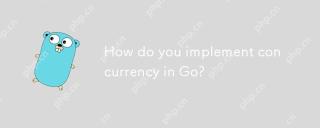
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
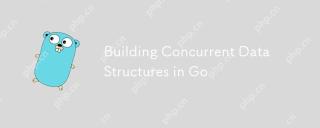
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
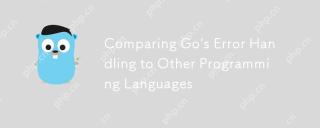
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Linux new version
SublimeText3 Linux latest version
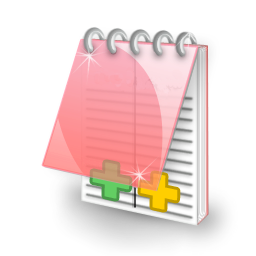
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
