Exploring Polymorphism in Go Language
In object-oriented programming, polymorphism allows objects to exhibit different behaviors based on their class. But in Go, the concept of polymorphism is not implemented in the traditional sense. Let's delve into the reasons behind this and explore how to achieve similar functionality in Go.
Why Go Lacks Traditional Polymorphism
Go is not a traditional object-oriented language. It adopts a different approach by using:
- Composition: Objects composed of other objects or interfaces.
- Interfaces: Contracts that define methods and behaviors for a specific type.
Unlike in object-oriented languages, Go does not support method overriding or virtual methods. This allows Go to maintain a higher level of type safety.
Implementing Polymorphism Using Composition and Interfaces
To achieve polymorphism-like behavior in Go, we can employ the following techniques:
- Create a Common Interface: Define an interface that represents the common behaviors or methods that you want your derived types to implement.
- Implement the Interface: Implement the interface's methods in your derived types, each providing its own unique implementation.
- Use Composition: Compose your derived types using the common interface as a field. This allows you to treat all derived types as instances of the common interface.
Example:
<code class="go">package main import "fmt" // Common interface type Foo interface { printFoo() } // Derived type with unique implementation type FooImpl struct{} func (f FooImpl) printFoo() { fmt.Println("Print Foo Impl") } // Derived type composed using the common interface type Bar struct { FooImpl } // Function returning the common interface func getFoo() Foo { return Bar{} } func main() { fmt.Println("Hello, playground") b := getFoo() b.printFoo() }</code>
In this example, Foo is the common interface, FooImpl is the derived type with its own implementation, and Bar is a derived type composed using FooImpl. The getFoo() function returns an instance of the Foo interface, allowing us to treat different derived types as one interface type.
This approach provides a form of polymorphism in Go by enabling us to handle different derived types as instances of a common interface.
The above is the detailed content of How Does Go Achieve Polymorphism Without Traditional Mechanisms?. For more information, please follow other related articles on the PHP Chinese website!
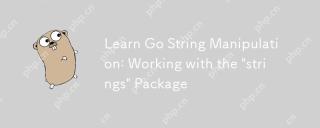
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
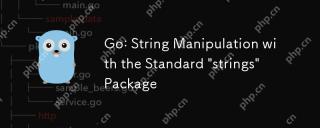
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
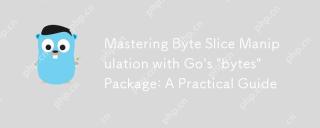
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
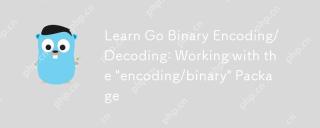
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
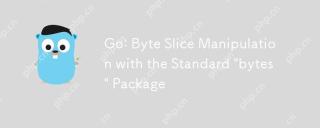
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
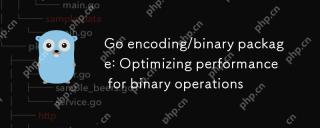
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
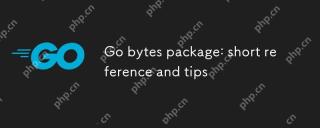
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
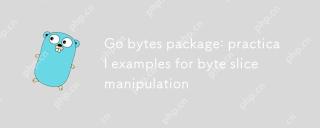
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
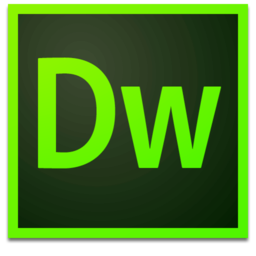
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
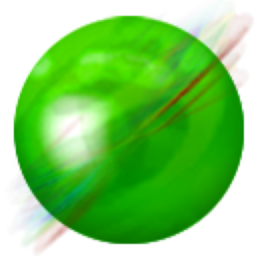
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
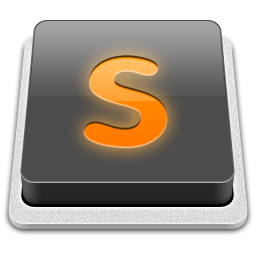
SublimeText3 Mac version
God-level code editing software (SublimeText3)
