Custom Helper Methods in Laravel without Facades
In Laravel, helper methods like myCustomMethod() are widely used for extending application functionality. Traditional methods involve creating a Facade, but this article presents an alternative approach for creating helper methods that seamlessly integrate with Laravel's native helpers.
Creating a Helper File
To commence, establish a file named helpers.php in any directory within your project. Within this file, define custom helper functions:
<code class="php">if (!function_exists('myCustomHelper')) { function myCustomHelper() { return 'Hey, it's working!'; } }</code>
Autoloading the Helper File
To make these helpers accessible throughout the application, modify your app's composer.json file. Under the autoload section, add the path to the helper file within the files array:
<code class="json">"autoload": { .... "files": [ "app/someFolder/helpers.php" ] },</code>
Running Composer Dumpauto
Execute the following command to update the composer autoloader cache:
composer dumpauto
Utilizing Custom Helper Methods
Once these steps are complete, your custom helper methods are ready for use throughout your Laravel application, just like the built-in Laravel helpers:
<code class="php">myCustomMethod(); // Will return 'Hey, it's working!'</code>
This approach allows for the creation of custom helper methods without introducing Facades, maintaining a clean and consistent coding style. Additionally, it aligns with Laravel's design philosophy of organizing application code into logical and maintainable structures.
The above is the detailed content of How to Create Custom Helper Methods in Laravel Without Facades?. For more information, please follow other related articles on the PHP Chinese website!
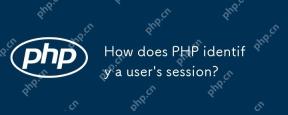
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
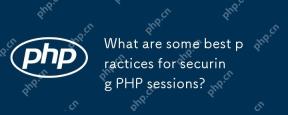
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
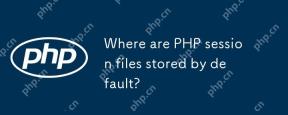
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita
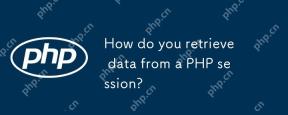
ToretrievedatafromaPHPsession,startthesessionwithsession_start()andaccessvariablesinthe$_SESSIONarray.Forexample:1)Startthesession:session_start().2)Retrievedata:$username=$_SESSION['username'];echo"Welcome,".$username;.Sessionsareserver-si
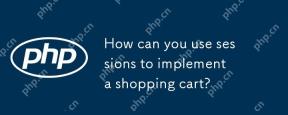
The steps to build an efficient shopping cart system using sessions include: 1) Understand the definition and function of the session. The session is a server-side storage mechanism used to maintain user status across requests; 2) Implement basic session management, such as adding products to the shopping cart; 3) Expand to advanced usage, supporting product quantity management and deletion; 4) Optimize performance and security, by persisting session data and using secure session identifiers.
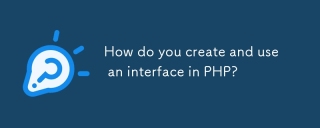
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
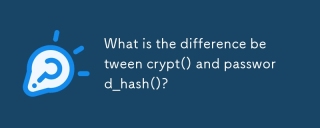
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
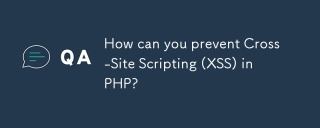
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
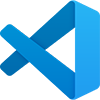
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
