How to Get Precisely Truncated Float Values?
Truncating float values, or removing digits beyond a specified number of decimal places, is a common operation in programming. However, achieving accurate truncation without rounding can be challenging due to the limitations of floating-point representation.
Understanding the Issue
Consider the example:
1.923328437452 -> 1.923
A naive approach would be to convert the float to a string and truncate it, but this can lead to errors due to the imprecise nature of floating-point representations. Different floating-point values may have the same binary representation, resulting in ambiguous truncation.
The Recommended Method
For Python versions 2.7 and above, the following function provides accurate truncation without rounding:
def truncate(f, n): '''Truncates/pads a float f to n decimal places without rounding''' s = '{}'.format(f) if 'e' in s or 'E' in s: return '{0:.{1}f}'.format(f, n) i, p, d = s.partition('.') return '.'.join([i, (d+'0'*n)[:n]])
Explanation
The core of this method is to convert the float to a string using '{}'.format(f) and truncate the string to the desired number of decimal places. For values in scientific notation ('e' or 'E'), the '{0:.{1}f}'.format(f, n) syntax is used to specify the precision.
Handling Special Cases
In rare cases, floats very close to round numbers may still be truncated incorrectly. For such cases, specify a higher precision to ensure that rounding during string conversion does not affect the result.
Alternatives for Older Python Versions
For Python versions below 2.7, a less accurate method involves rounding the float to 12 decimal places before truncation:
def truncate(f, n): '''Truncates/pads a float f to n decimal places without rounding''' s = '%.12f' % f i, p, d = s.partition('.') return '.'.join([i, (d+'0'*n)[:n]])
Conclusion
This code provides a robust solution for truncating float values without rounding, ensuring accurate truncation for a wide range of inputs.
The above is the detailed content of How to Precisely Truncate Float Values Without Rounding?. For more information, please follow other related articles on the PHP Chinese website!
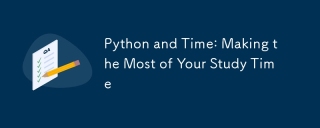
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
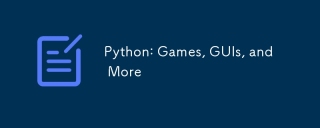
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
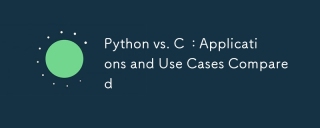
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
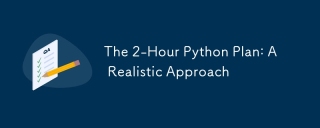
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
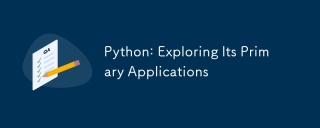
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
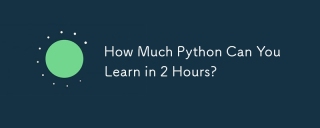
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
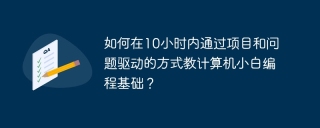
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
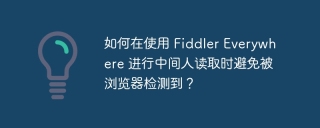
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
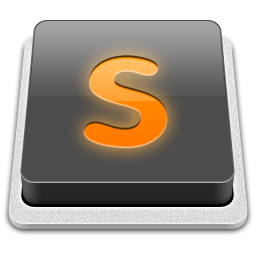
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
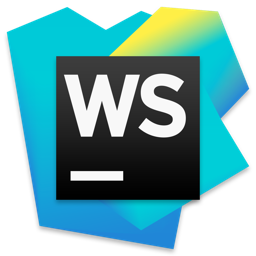
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
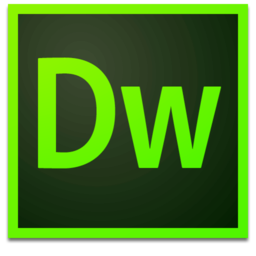
Dreamweaver Mac version
Visual web development tools