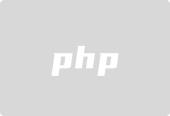
Q: How do you reverse a linked list?
- Answer: Reversing a linked list involves changing the direction of its pointers so that the list starts from the last element and ends at the first.
- Example:
Input: 1 -> 2 -> 3 -> 4 -> null
Output: 4 -> 3 -> 2 -> 1 -> null
Q: How do you perform binary search on a sorted array?
- Answer: Binary search divides the array in half repeatedly, checking if the middle element matches the target.
- Example:
Input: Array [1, 3, 5, 7, 9], Target = 7
Output: 3 (index of 7)
- Solution Approach: Check the middle element; if it’s the target, return the index. If the target is smaller, search the left half; if it is larger, search the right half.
Q: How do you find the first unique character in a string?
- Answer: To find the first unique character, count each character’s occurrences and identify the first one that appears only once.
- Example:
Input: "Swiss"
Output: "w"
- Solution Approach: Use a hash map to store each character’s frequency, then iterate through the string to find the first character with a count of 1.
Q: How do you detect a cycle in a linked list?
- Answer: To detect a cycle in a linked list, use two pointers (slow and fast). If there’s a cycle, the fast pointer will eventually meet the slow pointer.
- Example:
Input: 1 -> 2 -> 3 -> 4 -> 2 (cycle)
Output: True (cycle exists)
- Approach: Use Floyd’s Cycle Detection algorithm. Move the fast pointer two steps and the slow pointer one step. If they meet, there’s a cycle.
The above is the detailed content of Most Commonly Asked DSA Interview Questions. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn