


How Can We Implement Comparison Functionality in Go Without Operator Overloading?
The Comparable Interface: Implementing Comparison Functionality
The task at hand revolves around comparing values inserted into a linked list to maintain sorted insertion. In Go, where operator overloading is not supported, we need a mechanism to ensure that the Value field of the Element struct can be compared using the less-than operator (
A possible solution involves creating a Comparable interface. However, Go does not provide a dedicated interface to handle comparisons. Instead, we can emulate the desired functionality through a custom Compare method within a user-defined interface.
Conceptualizing the Comparable Interface
Consider the following simplified Comparable interface and its corresponding Compare method:
<code class="go">type Comparable interface { Compare(x Comparable) bool }</code>
This interface requires that any type implementing Comparable have a Compare method that takes another Comparable instance as input and returns a boolean value indicating the comparison result.
Implementing Comparable for Custom Types
To ensure that the Element struct can be compared, we can implement the Compare method for a custom type wrapping the Value field:
<code class="go">type ComparableValue struct { Value interface{} } func (c ComparableValue) Compare(other ComparableValue) bool { // Perform comparison logic based on the underlying Value field }</code>
By wrapping the Value field within a ComparableValue struct, we can implement the Compare method specific to the desired comparison logic.
Utilizing the Comparable Interface
With the ComparableValue type and its Compare method, we can modify the Element struct as follows:
<code class="go">type Element struct { next, prev *Element Value ComparableValue }</code>
In the Add method of the linked list, we can now call the Compare method to check for the appropriate insertion point:
<code class="go">for { if this.next.Value.Compare(val) <p>This approach allows us to implement comparison functionality without relying on operator overloading or built-in Comparable interfaces, making it suitable for custom types that require specific comparison logic.</p></code>
The above is the detailed content of How Can We Implement Comparison Functionality in Go Without Operator Overloading?. For more information, please follow other related articles on the PHP Chinese website!
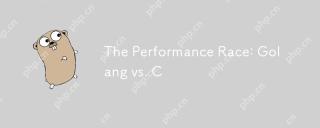
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
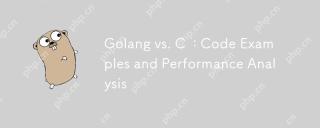
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
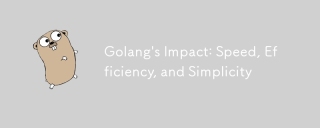
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
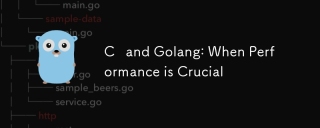
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
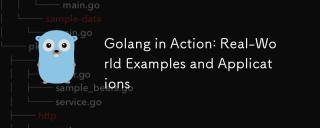
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
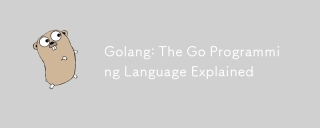
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
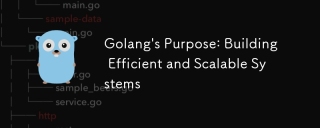
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
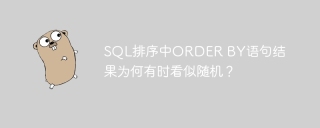
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
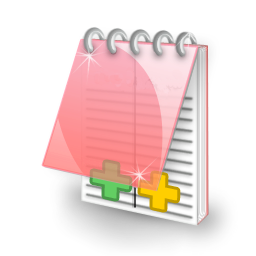
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
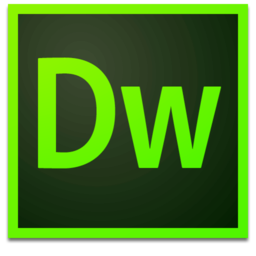
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor