


Customized Unmarshaling of JSON Data into Specific Structs
In Go, unmarshaling JSON data into predefined structs is a common task. However, this process can become more complex when you need to further customize the unmarshaled data structure. This article explores an approach to tailor the unmarshaling process to specific requirements.
Let's consider the following JSON data:
<code class="json">b := []byte(`{"Asks": [[21, 1], [22, 1]] ,"Bids": [[20, 1], [19, 1]]}`)</code>
Your initial approach involved defining a struct Message with two fields, Asks and Bids, each holding an array of floats. However, you seek a more specialized structure where these arrays contain structs of type Order instead of simple floats. The desired data structure looks like:
<code class="go">type Message struct { Asks []Order `json:"Bids"` Bids []Order `json:"Asks"` } type Order struct { Price float64 Volume float64 }</code>
To achieve this customization, we can leverage Go's json.Unmarshaler interface. By implementing this interface on the Order struct, we provide a custom unmarshaling logic that converts the JSON array elements into Order structs.
<code class="go">func (o *Order) UnmarshalJSON(data []byte) error { var v [2]float64 if err := json.Unmarshal(data, &v); err != nil { return err } o.Price = v[0] o.Volume = v[1] return nil }</code>
This implementation specifies that the Order type should be decoded from a 2-element array of floats, where the first element represents the price and the second element represents the volume.
Using this custom unmarshaling logic, you can now effectively unmarshal the JSON data into the desired struct Message.
<code class="go">m := new(Message) err := json.Unmarshal(b, &m) fmt.Println(m.Asks[0].Price)</code>
With the custom UnmarshalJSON implementation, this code will print the price of the first ask order. This customized unmarshaling approach provides a flexible way to tailor data unmarshaling to specific requirements in Go.
The above is the detailed content of How to Customize Unmarshaling of JSON Data into Specific Structs in Go?. For more information, please follow other related articles on the PHP Chinese website!
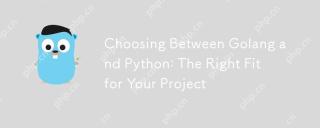
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
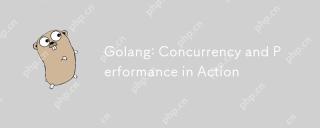
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
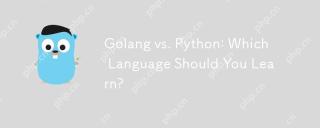
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
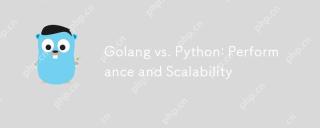
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
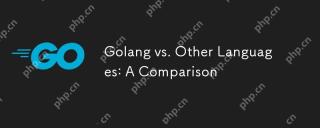
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
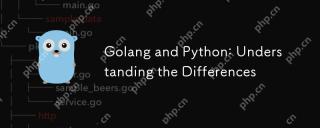
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
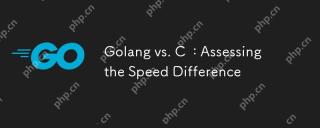
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
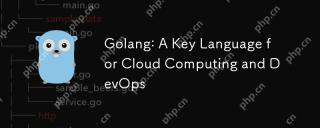
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
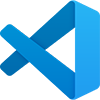
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
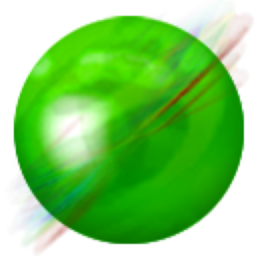
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment