Creating Multilingual Translated Routes in Laravel
In Laravel applications, you can create multilingual routes that depend on the selected language, allowing for a user-friendly navigation experience.
Step 1: Configure Language Directories and Translations
Create three separate language directories under app/lang (e.g., pl, en, fr). In each directory, create a file named routes.php that contains the translated route names:
<code class="php">// app/lang/pl/routes.php return array( 'contact' => 'kontakt', 'about' => 'o-nas' );</code>
<code class="php">// app/lang/en/routes.php return array( 'contact' => 'contact', 'about' => 'about-us' );</code>
<code class="php">// app/lang/fr/routes.php return array( 'contact' => 'contact-fr', 'about' => 'about-fr' );</code>
Step 2: Configure Language Settings
In app/config/app.php:
- Set 'locale' to your default language (e.g., 'pl').
- Include the list of alternative languages in 'alt_langs' (e.g., ['en', 'fr']).
- Leave 'locale_prefix' empty for the default language prefix.
<code class="php">'locale' => 'pl', 'alt_langs' => ['en', 'fr'], 'locale_prefix' => '',</code>
Step 3: Define Route Patterns and Language-Dependent Group
In app/routes.php:
- If the first segment of the URL matches an alternative language, update the locale and locale_prefix.
- Set route patterns based on the current language translations.
- Create a route group with the language prefix applied.
<code class="php">if (in_array(Request::segment(1), Config::get('app.alt_langs'))) { App::setLocale(Request::segment(1)); Config::set('app.locale_prefix', Request::segment(1)); } foreach(Lang::get('routes') as $k => $v) { Route::pattern($k, $v); } Route::group(array('prefix' => Config::get('app.locale_prefix')), function() { Route::get('/', function () { return "main page - ".App::getLocale(); }); Route::get('/{contact}/', function () { return "contact page ".App::getLocale(); }); Route::get('/{about}/', function () { return "about page ".App::getLocale(); }); });</code>
Step 4: Handle Unknown URLs
In app/start/global.php:
- Redirect any unknown URLs to the correct language prefix with a 301 status code:
<code class="php">App::missing(function() { return Redirect::to(Config::get('app.locale_prefix'), 301); });</code>
By implementing these steps, you will create dynamic routes that adapt to the selected language, ensuring a seamless user experience across multiple languages.
The above is the detailed content of How to Create Multilingual Translated Routes in Laravel?. For more information, please follow other related articles on the PHP Chinese website!
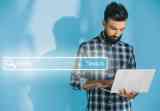
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
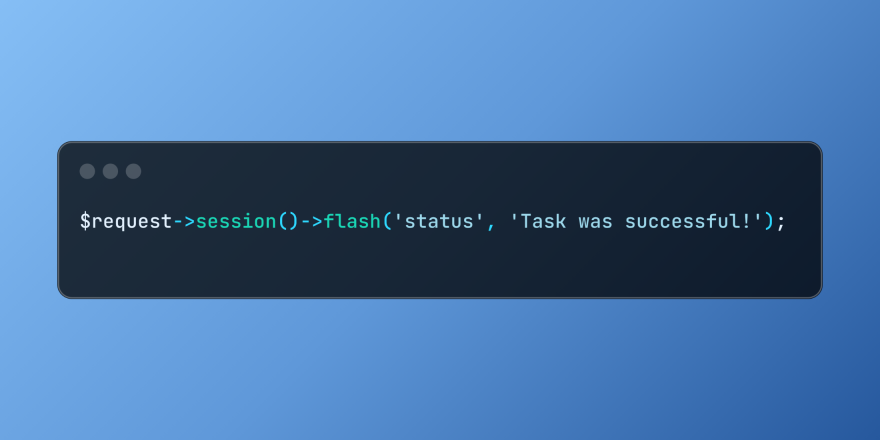
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
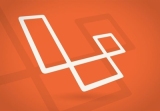
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
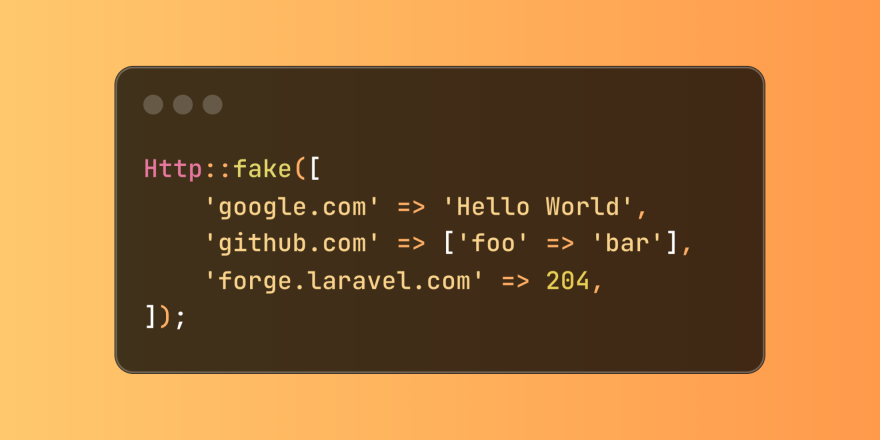
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
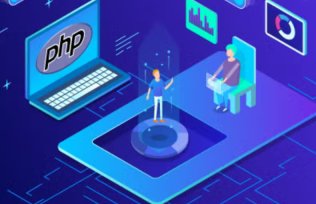
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
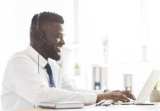
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
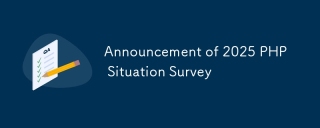
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
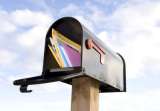
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
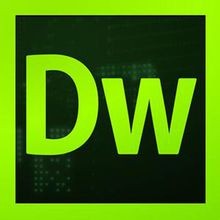
Dreamweaver CS6
Visual web development tools
