


How Can I Write Subprocess Output to Both the Terminal and Files Simultaneously in Python?
Writing Process Output to Terminal and Files Simultaneously with Python's subprocess
When executing multiple executables using subprocess.call(), it's desirable to have output displayed in both the terminal and a designated file. However, the default behavior only allows output redirection to either file or terminal.
To overcome this limitation, consider using Popen directly in conjunction with the stdout=PIPE argument. This will enable you to read output from the stdout attribute of the Popen object.
To achieve the desired behavior, employ the following steps:
- Create a tee() function: This function spawns a thread to forward input from one file to multiple output files.
- Implement a teed_call() function: This function emulates subprocess.call() but accepts additional stdout and stderr file arguments. It uses tee() to write output to both files and the terminal.
Here's an example of how to use these functions:
<code class="python">import sys from subprocess import Popen, PIPE from threading import Thread def tee(infile, *files): ... def teed_call(cmd_args, **kwargs): ... outf, errf = open("out.txt", "wb"), open("err.txt", "wb") assert not teed_call(["cat", __file__], stdout=None, stderr=errf) assert not teed_call(["echo", "abc"], stdout=outf, stderr=errf, bufsize=0) assert teed_call(["gcc", "a b"], close_fds=True, stdout=outf, stderr=errf)</code>
This code effectively writes output to both the files and the terminal concurrently for each executed command.
The above is the detailed content of How Can I Write Subprocess Output to Both the Terminal and Files Simultaneously in Python?. For more information, please follow other related articles on the PHP Chinese website!
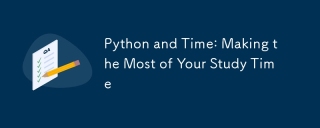
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
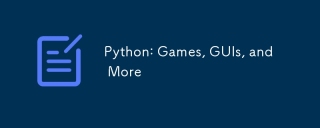
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
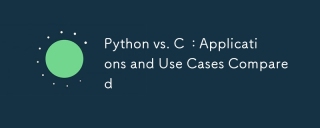
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
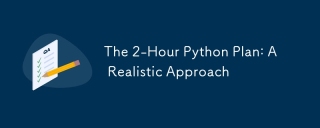
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
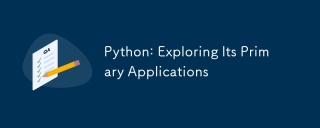
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
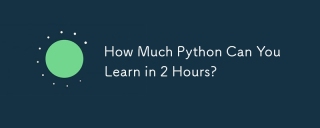
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
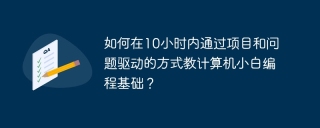
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
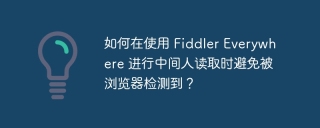
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
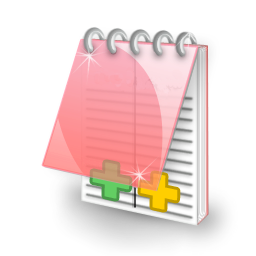
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
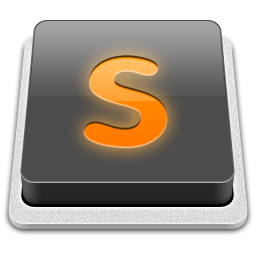
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.