


Why Does `console.log(content)` Log `undefined` When Using `fs.readFile` in Node.js?
Understanding the Asynchronous Nature of fs.readFile
When dealing with file system operations in Node.js, it's crucial to understand the asynchronous nature of fs.readFile. Unlike synchronous functions, asynchronous functions execute on a separate thread, allowing the main thread to continue without waiting for the operation to complete.
This can lead to unexpected results, as demonstrated in the following code snippet:
<code class="js">var content; fs.readFile('./Index.html', function read(err, data) { if (err) { throw err; } content = data; }); console.log(content); // Logs undefined, why?</code>
The issue arises because console.log is executed before the asynchronous callback function read has completed. As a result, content is still undefined at the time of logging.
Addressing the Asynchronicity
To resolve this issue, it's essential to account for the asynchronous nature of fs.readFile. There are several approaches to handle this:
- Nested Callbacks: Invoke the next step directly within the callback function.
- Separate Functions: Create a separate function to handle the next step after the asynchronous operation completes.
- Promises or Async/Await (ES2017): Employ promises or async/await syntax to handle asynchronous operations in a more structured way.
Example Code:
Using nested callbacks:
<code class="js">fs.readFile('./Index.html', function read(err, data) { if (err) { throw err; } console.log(data); });</code>
Using separate functions:
<code class="js">function readAndPrintFile() { fs.readFile('./Index.html', function read(err, data) { if (err) { throw err; } console.log(data); }); } readAndPrintFile();</code>
Using promises (ES2017):
<code class="js">const fsPromises = require('fs/promises'); fsPromises.readFile('./Index.html') .then((data) => console.log(data)) .catch((err) => console.error(err));</code>
The above is the detailed content of Why Does `console.log(content)` Log `undefined` When Using `fs.readFile` in Node.js?. For more information, please follow other related articles on the PHP Chinese website!
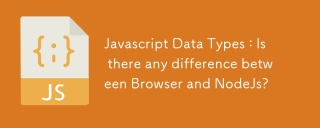
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
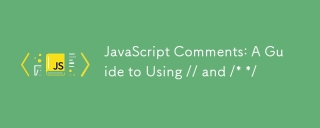
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
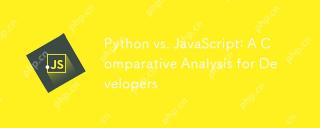
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
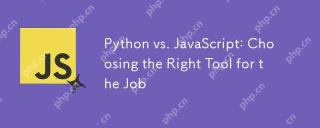
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
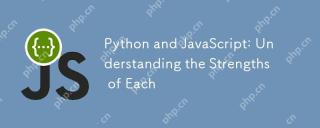
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
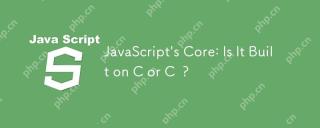
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
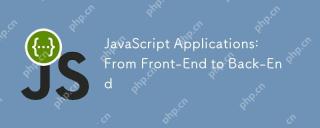
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
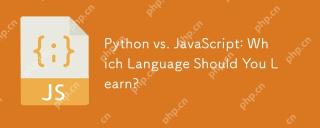
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
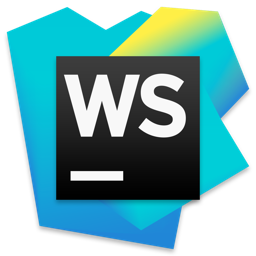
WebStorm Mac version
Useful JavaScript development tools
