Dynamically Switch UI Elements Based on Combo Box Selection
In a dialog box, you need to display specific sets of controls based on the selection made in a combo box. This is achieved by using multiple layers and switching between them when the combo box is checked or unchecked.
CardLayout for Control Layering
The CardLayout is an effective solution for this scenario. It allows you to have multiple panels, referred to as "cards," and display only one at a time by changing the layout.
The following code snippet demonstrates how to implement this:
<code class="java">import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.*; public class CardBoxExample { private static final CardLayout cardLayout = new CardLayout(); private static final JPanel cards = new JPanel(cardLayout); private static final JComboBox<string> combo = new JComboBox(); public static void main(String[] args) { // Create the cards JPanel panel1 = new JPanel(); panel1.setBackground(Color.RED); JPanel panel2 = new JPanel(); panel2.setBackground(Color.GREEN); cards.add(panel1, "Panel 1"); cards.add(panel2, "Panel 2"); // Add the combo box JPanel control = new JPanel(); combo.addItem("Panel 1"); combo.addItem("Panel 2"); combo.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { cardLayout.show(cards, combo.getSelectedItem().toString()); } }); control.add(combo); // Create the frame and add the components JFrame frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(cards, BorderLayout.CENTER); frame.add(control, BorderLayout.SOUTH); frame.pack(); frame.setVisible(true); } }</string></code>
When the combo box selection changes, the ActionListener updates the layout of the cards panel to display the selected card. This allows you to seamlessly transition between different groups of controls based on user input.
The above is the detailed content of How to Dynamically Switch UI Elements Based on Combo Box Selection?. For more information, please follow other related articles on the PHP Chinese website!
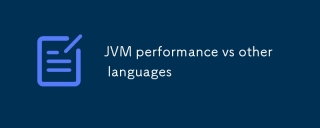
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
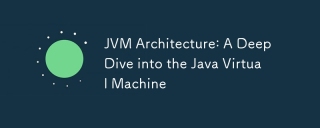
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
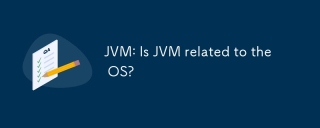
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
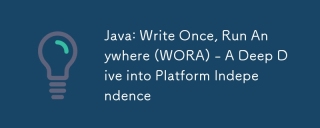
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
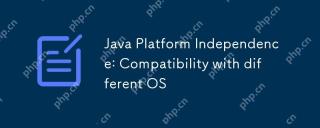
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
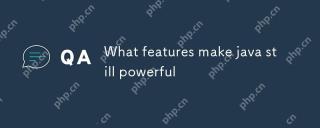
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
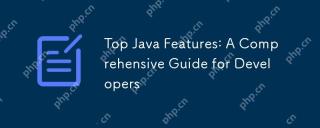
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
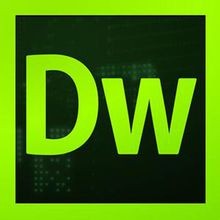
Dreamweaver CS6
Visual web development tools
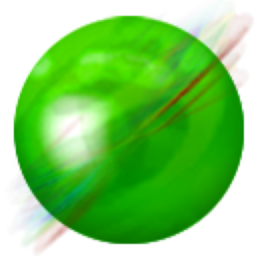
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
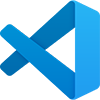
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
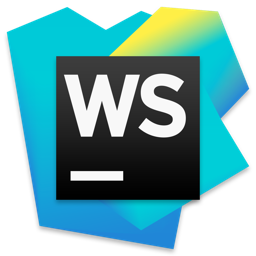
WebStorm Mac version
Useful JavaScript development tools
