


How to Access Variable Data from a Class
Problem Summary
You have multiple pages of widgets collecting user input in a multi-page Tkinter application. Each page is instantiated as a class in the main application, but you're facing difficulty in obtaining the value of a StringVar from a widget in one page (PageOne) in another page (PageTwo).
Solution: Leveraging your Controller
Utilizing the controller, you can establish communication between pages. Firstly, save a reference to the controller within each page:
<code class="python">class PageOne(ttk.Frame): def __init__(self, parent, controller): self.controller = controller ... class PageTwo(ttk.Frame): def __init__(self, parent, controller): self.controller = controller ...</code>
Secondly, incorporate a method in the controller that retrieves a page based on its class name:
<code class="python">class MyApp(Tk): ... def get_page(self, classname): '''Returns an instance of a page given it's class name as a string''' for page in self.frames.values(): if str(page.__class__.__name__) == classname: return page return None</code>
With this method, pages can access each other by calling this function and gaining a reference to the target page. Subsequently, you can access the shared members of that page, like so:
<code class="python">class PageTwo(ttk.Frame): ... def print_it(self): page_one = self.controller.get_page("PageOne") value = page_one.some_entry.get() print ('The value stored in StartPage some_entry = %s' % value)</code>
Alternative Solution: Storing Data in the Controller
To avoid tight coupling between pages, consider storing shared data in the controller. This method allows pages to be independent of each other's internal designs:
<code class="python">class MyApp(Tk): def __init__(self): ... self.app_data = {"name": StringVar(), "address": StringVar(), ... }</code>
Modify pages to reference the controller when creating widgets:
<code class="python">class PageOne(ttk.Frame): def __init__(self, parent, controller): self.controller=controller ... self.some_entry = ttk.Entry(self, textvariable=self.controller.app_data["name"], ...) </code>
Finally, access data from the controller, eliminating the need for get_page:
<code class="python"> def print_it(self): value = self.controller.app_data["address"].get() ...</code>
The above is the detailed content of How to Access StringVar Data from One Tkinter Page to Another?. For more information, please follow other related articles on the PHP Chinese website!
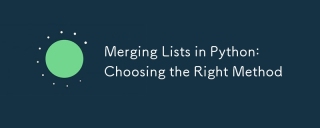
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
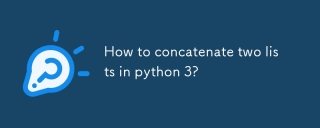
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
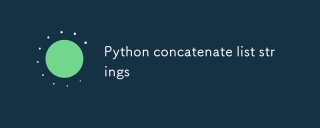
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
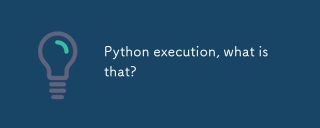
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
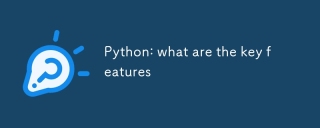
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
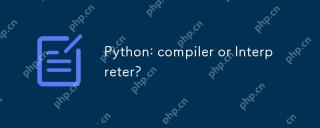
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
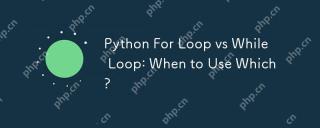
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
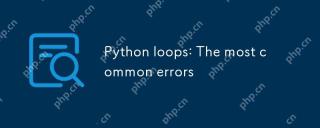
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
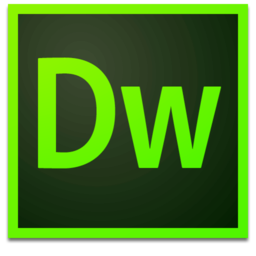
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
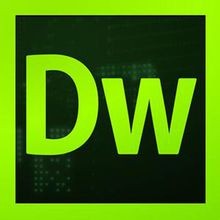
Dreamweaver CS6
Visual web development tools
