Injecting CSS into a Website via WebView in Android
When attempting to alter the appearance of a website loaded within a WebView, such as changing the background color of Google.com to red, by injecting a style.css file from the assets folder, unforeseen errors may arise.
The provided code fails to inject the style.css file because the WebView cannot directly access CSS files.
Solution:
To inject CSS into a website displayed in a WebView, a JavaScript-based approach must be utilized:
<code class="java">public class MainActivity extends ActionBarActivity { // Initialize WebView private WebView webView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Create WebView and enable JavaScript webView = new WebView(this); setContentView(webView); webView.getSettings().setJavaScriptEnabled(true); // Set WebViewClient for page load handling webView.setWebViewClient(new WebViewClient() { @Override public void onPageFinished(WebView view, String url) { // Inject CSS after page load injectCSS(); super.onPageFinished(view, url); } }); // Load the website webView.loadUrl("https://www.google.com"); } // Inject CSS method private void injectCSS() { try { // Retrieve style.css from assets folder InputStream inputStream = getAssets().open("style.css"); byte[] buffer = new byte[inputStream.available()]; inputStream.read(buffer); inputStream.close(); // Encode and insert CSS into HTML document head String encoded = Base64.encodeToString(buffer, Base64.NO_WRAP); webView.loadUrl("javascript:(function() {" + "var parent = document.getElementsByTagName('head').item(0);" + "var style = document.createElement('style');" + "style.type = 'text/css';" + "style.innerHTML = window.atob('" + encoded + "');" + "parent.appendChild(style)" + "})()"); } catch (Exception e) { e.printStackTrace(); } } // ... (Other methods and menus) }</code>
This improved code enables the injection of CSS by utilizing JavaScript. It reads the style.css file from the assets folder, encodes it into Base64, and embeds it into the HTML document head, allowing for the manipulation of website styles.
The above is the detailed content of How to Inject CSS into a Website Displayed in an Android WebView?. For more information, please follow other related articles on the PHP Chinese website!
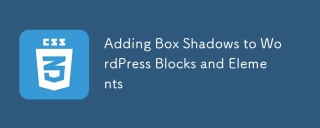
The CSS box-shadow and outline properties gained theme.json support in WordPress 6.1. Let's look at a few examples of how it works in real themes, and what options we have to apply these styles to WordPress blocks and elements.
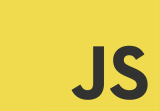
This tutorial demonstrates creating professional-looking JavaScript forms using the Smart Forms framework (note: no longer available). While the framework itself is unavailable, the principles and techniques remain relevant for other form builders.
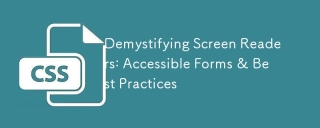
This is the 3rd post in a small series we did on form accessibility. If you missed the second post, check out "Managing User Focus with :focus-visible". In
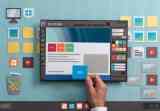
Building an inline text editor isn't trivial. The process starts by making the target element editable, handling potential SyntaxError exceptions along the way. Creating Your Editor To build this editor, you'll need to dynamically modify the content
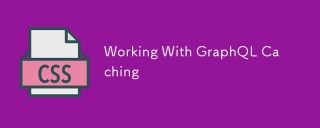
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
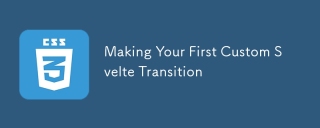
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
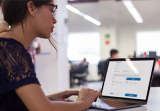
This article explores the top PHP form builder scripts available on Envato Market, comparing their features, flexibility, and design. Before diving into specific options, let's understand what a PHP form builder is and why you'd use one. A PHP form
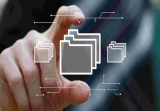
This tutorial guides you through building a file upload system using Node.js, Express, and Multer. We'll cover single and multiple file uploads, and even demonstrate storing images in a MongoDB database for later retrieval. First, set up your projec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
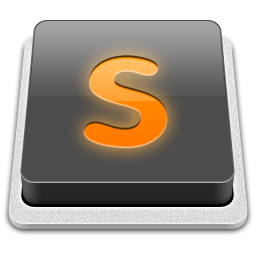
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
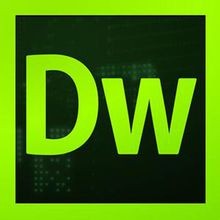
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
