This tutorial guides you through building a file upload system using Node.js, Express, and Multer. We'll cover single and multiple file uploads, and even demonstrate storing images in a MongoDB database for later retrieval.
First, set up your project:
mkdir upload-express cd upload-express npm init -y npm install express multer mongodb file-system --save touch server.js mkdir uploads
Next, create your server.js
file. This will handle the file uploads and server logic. Initially, we'll set up a basic Express app:
const express = require('express'); const multer = require('multer'); const app = express(); app.get('/', (req, res) => { res.json({ message: 'WELCOME' }); }); app.listen(3000, () => console.log('Server started on port 3000'));
Now, let's configure Multer to handle file uploads to disk:
//server.js (add this to your existing server.js) var storage = multer.diskStorage({ destination: function (req, file, cb) { cb(null, 'uploads') }, filename: function (req, file, cb) { cb(null, file.fieldname + '-' + Date.now()) } }); var upload = multer({ storage: storage });
Handling File Uploads
Single File Upload
Create an endpoint to handle single file uploads. Remember to create a corresponding <form></form>
with a file input in your index.html
(not shown here, but should use a POST request to /uploadfile
).
//server.js (add this to your existing server.js) app.post('/uploadfile', upload.single('myFile'), (req, res, next) => { const file = req.file; if (!file) { const error = new Error('Please upload a file'); error.httpStatusCode = 400; return next(error); } res.send(file); });
Multiple File Uploads
This endpoint handles uploading multiple files (up to 12 in this example):
//server.js (add this to your existing server.js) app.post('/uploadmultiple', upload.array('myFiles', 12), (req, res, next) => { const files = req.files; if (!files) { const error = new Error('Please choose files'); error.httpStatusCode = 400; return next(error); } res.send(files); });
Image Upload to MongoDB
To store images in MongoDB, we'll need to install the mongodb
package:
npm install mongodb --save
Then, add the MongoDB connection and image handling logic to server.js
. (Note: Error handling and connection details are omitted for brevity. Replace placeholders like <your_mongodb_connection_string></your_mongodb_connection_string>
with your actual connection string).
const { MongoClient } = require('mongodb'); const fs = require('file-system'); // For file system operations // ... (previous code) ... const client = new MongoClient("<your_mongodb_connection_string>"); // ... (rest of your code) ... app.post('/uploadImage', upload.single('myImage'), async (req, res) => { try { await client.connect(); const db = client.db('your_database_name'); const imageBuffer = fs.readFileSync(req.file.path); const result = await db.collection('images').insertOne({ image: { filename: req.file.filename, buffer: imageBuffer } }); res.send(result); } catch (error) { console.error(error); res.status(500).send("Error uploading image"); } finally { await client.close(); } }); app.get('/photos', async (req, res) => { try { await client.connect(); const db = client.db('your_database_name'); const result = await db.collection('images').find().toArray(); const imgArray = result.map(element => element._id); res.send(imgArray); } catch (error) { console.error(error); res.status(500).send("Error retrieving images"); } finally { await client.close(); } }); app.get('/photo/:id', async (req, res) => { try { await client.connect(); const db = client.db('your_database_name'); const result = await db.collection('images').findOne({ _id: new ObjectId(req.params.id) }); res.contentType('image/jpeg'); // Adjust content type as needed res.send(result.image.buffer); } catch (error) { console.error(error); res.status(500).send("Error retrieving image"); } finally { await client.close(); } }); // ... (rest of your code) ...</your_mongodb_connection_string>
Remember to install the mongodb
and file-system
packages. Also replace placeholders with your database name and connection string. This improved example includes error handling and asynchronous operations for better robustness.
This enhanced tutorial provides a more complete and robust solution for handling file uploads in a Node.js application. Remember to adapt the code to your specific needs and environment. Always prioritize security best practices when handling file uploads in production.
The above is the detailed content of File Upload With Multer in Node.js and Express. For more information, please follow other related articles on the PHP Chinese website!
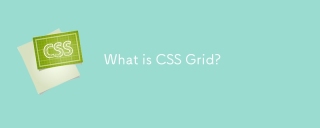
CSS Grid is a powerful tool for creating complex, responsive web layouts. It simplifies design, improves accessibility, and offers more control than older methods.
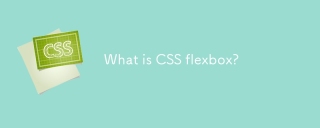
Article discusses CSS Flexbox, a layout method for efficient alignment and distribution of space in responsive designs. It explains Flexbox usage, compares it with CSS Grid, and details browser support.
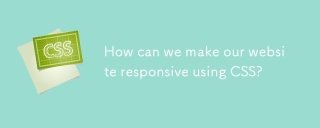
The article discusses techniques for creating responsive websites using CSS, including viewport meta tags, flexible grids, fluid media, media queries, and relative units. It also covers using CSS Grid and Flexbox together and recommends CSS framework
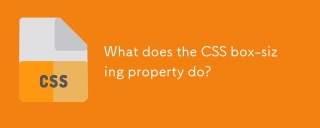
The article discusses the CSS box-sizing property, which controls how element dimensions are calculated. It explains values like content-box, border-box, and padding-box, and their impact on layout design and form alignment.
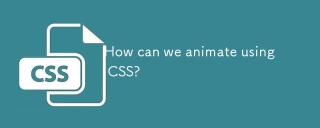
Article discusses creating animations using CSS, key properties, and combining with JavaScript. Main issue is browser compatibility.
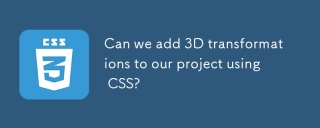
Article discusses using CSS for 3D transformations, key properties, browser compatibility, and performance considerations for web projects.(Character count: 159)
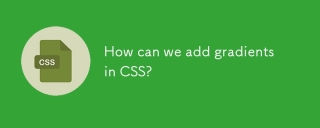
The article discusses using CSS gradients (linear, radial, repeating) to enhance website visuals, adding depth, focus, and modern aesthetics.
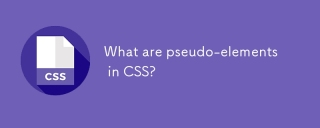
Article discusses pseudo-elements in CSS, their use in enhancing HTML styling, and differences from pseudo-classes. Provides practical examples.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
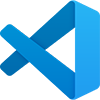
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
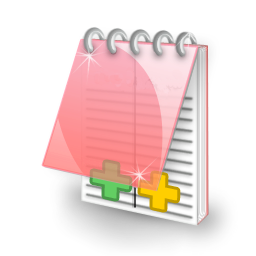
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
