Downloading Files After POSTing Data using FastAPI
When developing web applications that need to receive user input, process it, and then provide a file for download, the ability to properly facilitate this exchange is crucial. FastAPI provides a robust framework for building such applications, and this guide will explore how to effectively download a file after processing POSTed data.
Implementing the Function
First, define an endpoint in your FastAPI application to handle the file download request. This endpoint will receive the file path and return the file as a response. A sample implementation using the FastAPI FileResponse class:
<code class="python">from fastapi import FastAPI, FileResponse, Request from fastapi.responses import FileResponse app = FastAPI() @app.post('/download-file') async def download_file(request: Request, user_id: int = Form(...)): file_path = 'path/to/file.mp3' return FileResponse(file_path, media_type='audio/mp3', filename='output.mp3')</code>
In this code, the file_path variable should be replaced with the actual path to the file that needs to be downloaded. The media_type and filename parameters specify the type of file and the name under which it will be downloaded.
Using HTML to Trigger the Download
After defining the endpoint, incorporate an HTML form into your frontend that triggers a POST request to the /download-file endpoint and provides the necessary user_id parameter:
<code class="html"><form action="/download-file" method="post"> <input type="text" name="user_id" placeholder="Enter user ID"> <input type="submit" value="Download File"> </form></code>
When the form is submitted, a POST request with the specified user_id will be sent to the /download-file endpoint, which will then process the request and return the file for download.
Handling Large File Downloads
If the file to be downloaded is particularly large, consider using the StreamingResponse class in FastAPI:
<code class="python">from fastapi import FastAPI, Response, StreamingResponse @app.post('/stream-large-file') async def download_large_file(request: Request, user_id: int = Form(...)): file_path = 'path/to/large_file.mp3' async def iter_file(): with open(file_path, 'rb') as f: while chunk := f.read(1024): yield chunk return StreamingResponse(iter_file(), media_type='audio/mp3', filename='output.mp3')</code>
Here, the iter_file function reads the file in chunks to reduce memory consumption and facilitates the streaming of the file.
Conclusion
By following the steps outlined above, you can create FastAPI applications that seamlessly handle file downloads after POST operations. This empowers your applications to offer download functionality, enriching the user experience and making it easier to access generated files.
The above is the detailed content of How to Download Files After POSTing Data using FastAPI?. For more information, please follow other related articles on the PHP Chinese website!
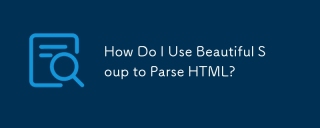
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
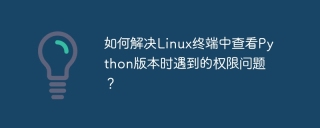
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
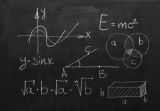
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
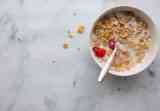
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
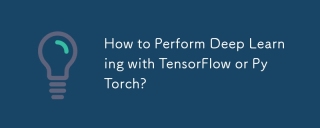
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
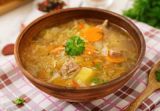
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
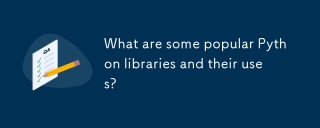
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
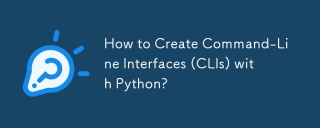
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
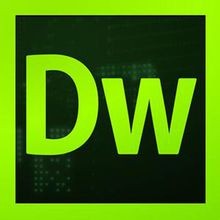
Dreamweaver CS6
Visual web development tools
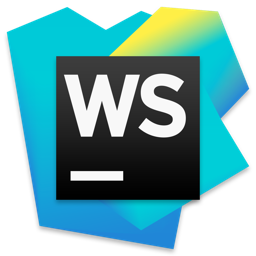
WebStorm Mac version
Useful JavaScript development tools