


Using Unsafe to Obtain a Byte Slice from a String without Copying
Go strings are immutable, meaning that converting them to byte slices involves a memory copy. This can potentially impact performance when working with large datasets. This article explores how to use unsafe to avoid this copy operation while emphasizing the critical aspects and limitations.
Background
The standard library function []byte(s) creates a copy of the string s. If memory consumption is a concern, it is desirable to obtain the byte slice without incurring this overhead.
Unsafe Conversion
Utilizing the unsafe package provides a way to achieve this goal. By casting the string value to a pointer to an array of bytes, we can access the underlying byte slice without creating a copy.
<code class="go">func unsafeGetBytes(s string) []byte { return (*[0x7fff0000]byte)(unsafe.Pointer( (*reflect.StringHeader)(unsafe.Pointer(&s)).Data), )[:len(s):len(s)] }</code>
Cautions
It is crucial to note that this approach carries inherent risks. Strings in Go are immutable, so modifying the byte slice obtained through unsafeGetBytes could result in unexpected behavior or even data corruption. Therefore, this technique should be used only in controlled internal environments where memory performance is paramount.
Handling Empty Strings
Note that the empty string ("") has no bytes, so its data field is indeterminate. If your code may encounter empty strings, explicitly checking for them is essential.
<code class="go">func unsafeGetBytes(s string) []byte { if s == "" { return nil // or []byte{} } return (*[0x7fff0000]byte)(unsafe.Pointer( (*reflect.StringHeader)(unsafe.Pointer(&s)).Data), )[:len(s):len(s)] }</code>
Performance Considerations
While this conversion avoids the overhead of copying, it is essential to keep in mind that compression operations, such as the one you mentioned using gzipWriter, are computationally intensive. The potential performance gain from avoiding the memory copy may be negligible compared to the computation required for compression.
Alternative Approaches
Alternatively, the io.WriteString function can be leveraged to write strings to an io.Writer without invoking the copy operation. The function checks for the existence of the WriteString method on the io.Writer and invokes it if available.
Related Questions and Resources
For further exploration, consider the following resources:
- [Go GitHub Issue 25484](https://github.com/golang/go/issues/25484)
- [unsafe.String](https://pkg.go.dev/unsafe#String)
- [unsafe.StringData](https://pkg.go.dev/unsafe#StringData)
- [[]byte(string) vs []byte(*string)](https://stackoverflow.com/questions/23369632/)
- [What are the possible consequences of using unsafe conversion from []byte to string in go?](https://stackoverflow.com/questions/67306718/)
The above is the detailed content of How to Obtain a Byte Slice from a Go String without Copying Using `unsafe`?. For more information, please follow other related articles on the PHP Chinese website!
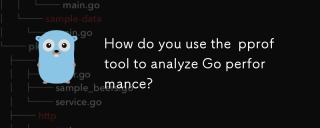
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
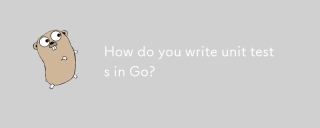
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
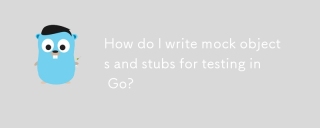
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
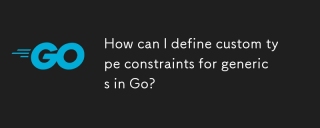
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
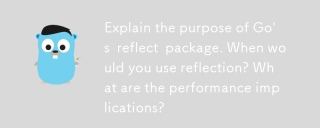
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
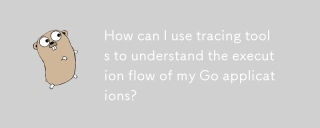
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
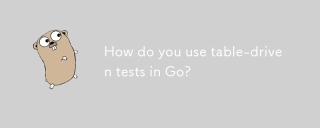
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
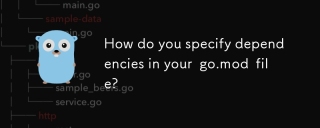
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
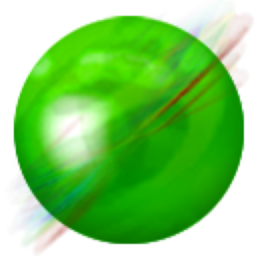
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
