Retrieving Pixel Color under Mouse on Canvas Using Mousemove
In web applications, it is often useful to obtain the RGB value of the pixel directly beneath the mouse cursor when it interacts with a canvas element. Here's how to achieve this in JavaScript:
Example:
Consider this HTML code:
<code class="html"><canvas id="canvas" width="200" height="200"></canvas> <div id="color-value"></div></code>
And the following JavaScript code:
<code class="js">const canvas = document.getElementById("canvas"); const context = canvas.getContext("2d"); // Set up an image on the canvas const img = new Image(); img.src = "path/to/image.jpg"; img.onload = function() { context.drawImage(img, 0, 0); }; // Add event listener for mouse movement canvas.addEventListener("mousemove", (e) => { const rect = canvas.getBoundingClientRect(); const x = e.clientX - rect.left; const y = e.clientY - rect.top; const pixelData = context.getImageData(x, y, 1, 1).data; const r = pixelData[0]; const g = pixelData[1]; const b = pixelData[2]; document.getElementById("color-value").innerHTML = `RGB: ${r}, ${g}, ${b}`; });</code>
In this example, the drawImage method is used to display an image on the canvas. When the mouse moves over the canvas, the mousemove event listener captures the current mouse position and calculates the pixel coordinates within the canvas using the getBoundingClientRect function. It then retrieves the pixel data using the getImageData method and displays the RGB values in the color-value div.
Additional Notes:
- The pixelData array returned by getImageData contains four values: red, green, blue, and alpha (transparency).
- The event listener can be modified to perform other actions, such as rendering a tooltip or updating a UI element.
- This technique can be extended to work with dynamic content on the canvas, such as drawn shapes or moving objects.
The above is the detailed content of How to Get the RGB Value of a Pixel Under the Mouse on a Canvas?. For more information, please follow other related articles on the PHP Chinese website!
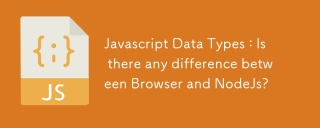
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
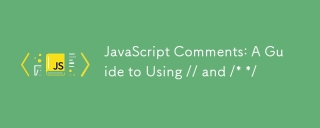
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
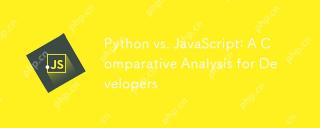
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
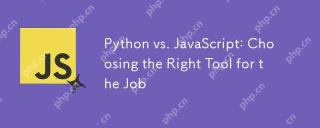
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
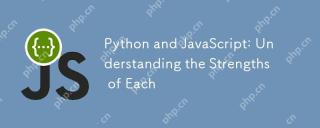
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
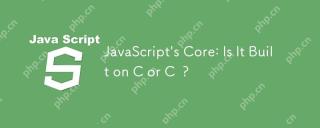
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
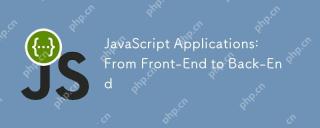
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
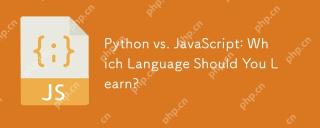
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
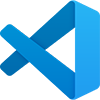
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
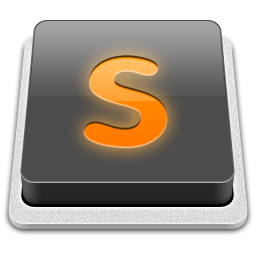
SublimeText3 Mac version
God-level code editing software (SublimeText3)
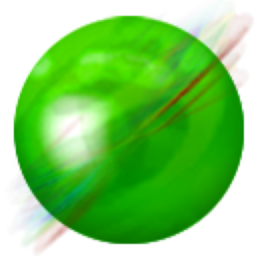
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
