


How to Efficiently Extract Values from Deeply Nested JSON Structures in Python?
Retrieving Nested Data from JSON Structures
When parsing complex JSON data, it can be challenging to extract specific values from deeply nested structures. This article provides a solution to this issue by outlining how to navigate nested data efficiently using Python's dictionary and list indexing syntax.
Understanding the Data Structure
Consider the following example JSON data:
<code class="python">my_json = { "name": "ns1:timeSeriesResponseType", "value": { "queryInfo": { "creationTime": 1349724919000, # ... other data } } }</code>
Extracting a Specific Value
To access the "creationTime" value, use the following syntax:
<code class="python">query_info = my_json["value"]["queryInfo"] creation_time = query_info["creationTime"]</code>
Breaking it down:
- my_json["value"] accesses the value of the "value" key in the outer dictionary (the parsed JSON data itself).
- query_info["creationTime"] accesses the value of the "creationTime" key in the "queryInfo" dictionary (which is obtained from my_json["value"]).
Therefore, creation_time will have the value 1349724919000.
Generalizing the Extraction Code
For nested data with variable levels of nesting, you can use a more general approach:
<code class="python">def get_nested_value(data, key_path): keys = key_path.split(".") value = data for key in keys: value = value[key] return value</code>
Usage:
<code class="python">creation_time = get_nested_value(my_json, "value.queryInfo.creationTime")</code>
This function takes a data dictionary and a key path (e.g., "value.queryInfo.creationTime") and iteratively accesses nested values until the desired value is obtained.
The above is the detailed content of How to Efficiently Extract Values from Deeply Nested JSON Structures in Python?. For more information, please follow other related articles on the PHP Chinese website!
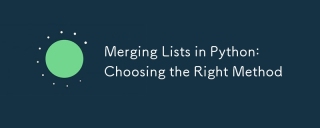
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
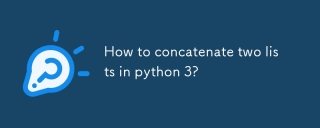
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
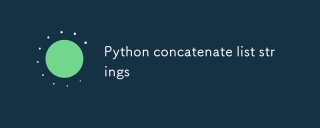
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
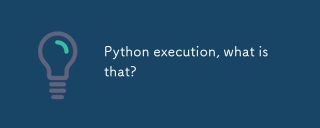
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
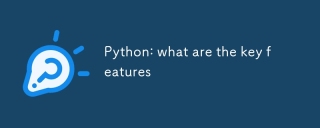
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
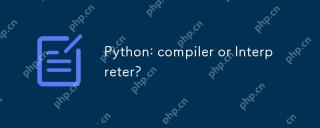
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
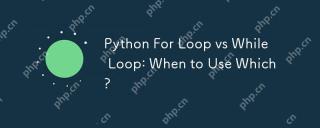
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
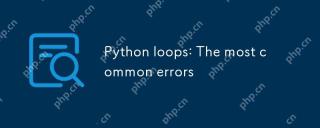
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
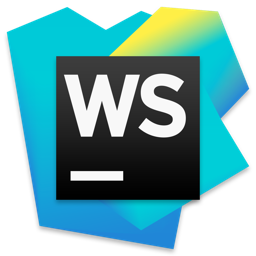
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
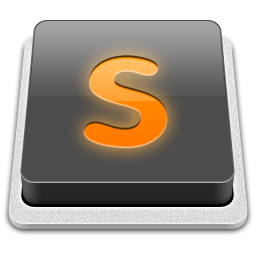
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
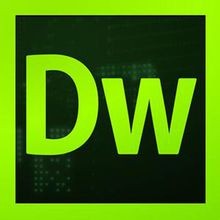
Dreamweaver CS6
Visual web development tools
