


Efficient Set-like Storage in JavaScript
In JavaScript, objects can mimic the functionality of sets to provide efficient storage and manipulation of unique, unordered string values. To achieve the desired properties—fast lookup, deletion, and addition—consider the following techniques:
Utilizing Objects
An object can serve as a rudimentary set by utilizing its keys to store properties. Initialize the object as empty, and add items by setting the property key to true. To check for membership, access the property using the key. Deletion and addition operations are straightforward, involving delete and property setting respectively.
<code class="javascript">// Initialize empty object var obj = {}; // Add items obj["key1"] = true; obj["key2"] = true; // Check membership if ("key1" in obj) { /* ... */ } // Delete an item delete obj["key1"]; // Add an item (if not present) if (!("key3" in obj)) { obj["key3"] = true; }</code>
ES6 Set Object (ES6 )
ES6 introduces a built-in Set object tailored for managing sets. It features:
- Fast checking for membership with .has()
- Deletion with .delete()
- Addition with .add()
<code class="javascript">// Initialize empty Set const mySet = new Set(); // Add items mySet.add("item1"); mySet.add("item2"); // Check membership if (mySet.has("item1")) { /* ... */ } // Delete an item mySet.delete("item1"); // Iterate over elements mySet.forEach((value) => console.log(`Element: ${value}`));</code>
Pre-built Set Objects
Various pre-built set objects exist for cross-browser compatibility:
- miniSet: A compact set implementation with core functionality.
- set2: A feature-rich set object with methods for complex operations.
- dictionary: Allows storage and retrieval of values associated with keys.
- objectSet: Maintains a set of objects with unique key generation.
Conclusion
The choice of approach depends on the complexity and compatibility requirements of your application. If performance is paramount and ES6 capabilities are available, the ES6 Set object is optimal. For ES5 environments, utilizing objects provides a viable alternative. Pre-built set objects offer flexibility and cross-browser compatibility.
The above is the detailed content of How can JavaScript efficiently store and manage unique, unordered string values?. For more information, please follow other related articles on the PHP Chinese website!
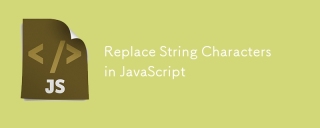
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
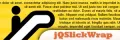
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
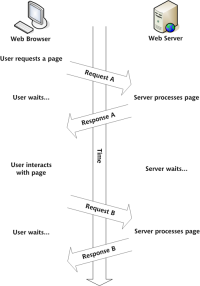
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
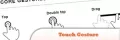
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
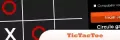
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
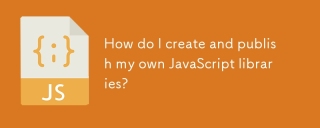
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
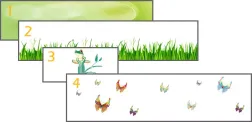
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
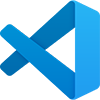
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
