


Detecting the Number of Physical Processors/Cores with Hyper-Threading Support
In multi-threaded applications that aim for maximum efficiency, knowing the number of physical processors or cores is crucial. Creating excessive threads can hinder performance, especially in scenarios where hyper-threading is supported.
Hyper-Threading Detection
To accurately determine the number of physical processors, you need to detect if hyper-threading is supported and enabled. Here's how you can do it:
- Identify CPU Vendor: Execute the CPUID instruction with function 0 to retrieve the CPU vendor (e.g., "GenuineIntel" or "AuthenticAMD").
- Check for Hyper-Threading (Intel): For Intel processors, check bit 28 in EDX from CPUID function 1. If it's set, hyper-threading is supported.
- Check for Hyper-Threading (AMD): For AMD processors, execute CPUID function 0x80000008 to obtain the number of cores in ECX[7:0]. If this number is greater than zero, hyper-threading is supported.
Determining Physical Core Count
Once hyper-threading support is detected, follow these steps to determine the number of physical cores:
- For Intel processors, execute CPUID function 4 and get the count from EAX[31:26] 1.
- For AMD processors, use the previously obtained ECX[7:0] value from CPUID function 0x80000008 and add 1.
Example Implementation
The following C program demonstrates the detection of hyper-threading and the number of physical cores:
<code class="cpp">#include <iostream> #include <string> using namespace std; void cpuID(unsigned i, unsigned regs[4]) { #ifdef _WIN32 __cpuid((int *)regs, (int)i); #else asm volatile ("cpuid" : "=a" (regs[0]), "=b" (regs[1]), "=c" (regs[2]), "=d" (regs[3]) : "a" (i), "c" (0)); #endif } int main(int argc, char *argv[]) { unsigned regs[4]; // ... (Code for vendor detection, feature check, and logical core count) // Hyper-Threading detection bool hyperThreads = cpuFeatures & (1 <p><strong>Conclusion</strong></p> <p>By following these steps, you can accurately detect the number of physical processors/cores while accounting for hyper-threading support. This information is invaluable for optimizing the performance of your multi-threaded applications.</p></string></iostream></code>
The above is the detailed content of How can I accurately determine the number of physical cores in my system, considering the presence of hyper-threading?. For more information, please follow other related articles on the PHP Chinese website!
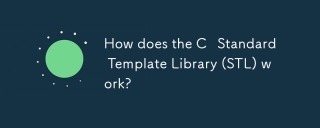
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
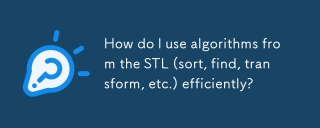
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
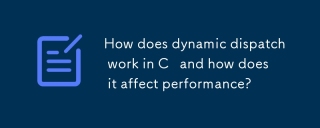
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
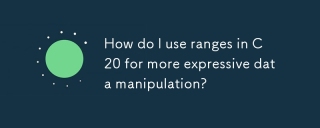
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
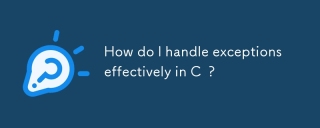
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
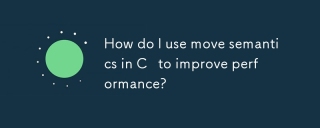
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
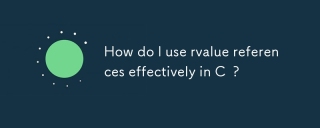
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
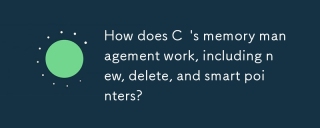
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
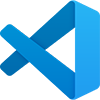
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
