The title could be: How to Switch Between JPanels in a JFrame Using CardLayout?
How Can I Transition Between JPanels within a JFrame?
Initial Question:
A beginner in Java components seeks assistance in switching between MenuPanel and GamePanel within a JFrame. The current code only creates a blank JFrame upon calling gameOn(), rather than initiating the game. Suggestions are welcomed to resolve this issue, taking into account the presence of a mouse listener in MenuPanel.
Solution:
Instead of employing the approach of removing and adding components, it is advisable to utilize a CardLayout. This allows for the seamless transition between panels without the need for repeated addition and removal.
Code Implementation:
<code class="java">CardLayout cardLayout = new CardLayout(); JPanel mainPanel = new JPanel(cardLayout); MenuPanel menu = new MenuPanel(); GamePanel game = new GamePanel(); mainPanel.add(menu, "menu"); mainPanel.add(game, "game"); ... public void gameOn() { cardLayout.show(mainPanel, "game"); }</code>
Explanation:
- When gameOn() is invoked, the MenuPanel will be pushed to the back and rendered as hidden, while the GamePanel will be brought to the front and displayed.
Example:
For a practical demonstration, consider the following code:
<code class="java">import java.awt.BorderLayout; import java.awt.CardLayout; import java.awt.Color; import java.awt.Dimension; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.SwingUtilities; public class GameFrame extends JFrame implements ActionListener{ CardLayout cardLayout; JPanel mainPanel; MenuPanel menu; GamePanel game; public GameFrame() { cardLayout = new CardLayout(); mainPanel = new JPanel(cardLayout); menu = new MenuPanel(); game = new GamePanel(); mainPanel.add(menu, "menu"); mainPanel.add(game, "game"); JButton goGame = new JButton("Go TO Game"); goGame.addActionListener(this); add(mainPanel); add(goGame, BorderLayout.SOUTH); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); pack(); setLocationByPlatform(true); setVisible(true); } @Override public void actionPerformed(ActionEvent e) { gameOn(); } public void gameOn() { cardLayout.show(mainPanel, "game"); } public static void main(String[] args) { SwingUtilities.invokeLater(new Runnable(){ @Override public void run() { GameFrame gameFrame = new GameFrame(); } }); } } class MenuPanel extends JPanel { public MenuPanel() { setBackground(Color.GREEN); add(new JLabel("Menu")); } @Override public Dimension getPreferredSize() { return new Dimension(300, 300); } } class GamePanel extends JPanel { public GamePanel() { setBackground(Color.BLUE); add(new JLabel("Game")); } @Override public Dimension getPreferredSize() { return new Dimension(300, 300); } }</code>
This implementation showcases the use of CardLayout to smoothly transition between the MenuPanel and GamePanel within a JFrame.
The above is the detailed content of The title could be: How to Switch Between JPanels in a JFrame Using CardLayout?. For more information, please follow other related articles on the PHP Chinese website!
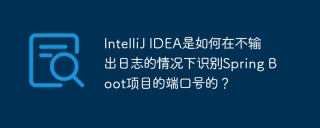
Start Spring using IntelliJIDEAUltimate version...
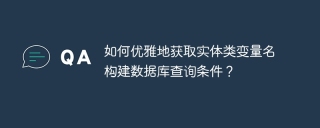
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
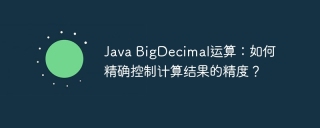
Java...
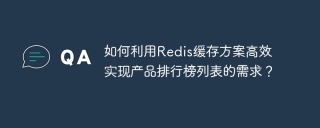
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
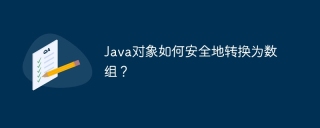
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
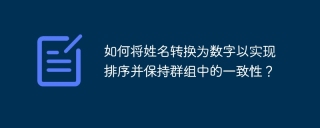
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
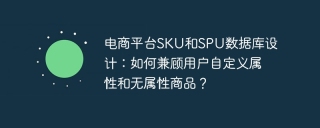
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
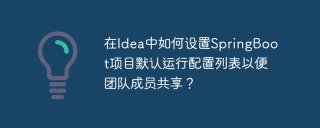
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
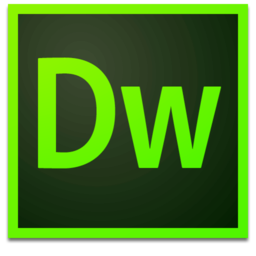
Dreamweaver Mac version
Visual web development tools
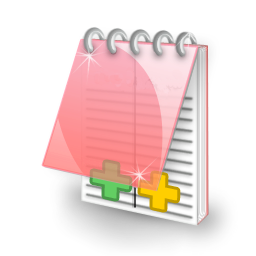
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor