TypeScript's type system is powerful, but its error messages can sometimes be cryptic and hard to understand. In this article, we'll explore a pattern that uses unconstructable types to create clear, descriptive compile-time exceptions. This approach helps prevent runtime errors by making invalid states unrepresentable with helpful error messages.
The Pattern: Unconstructable Types with Custom Messages
First, let's break down the core pattern:
// Create a unique symbol for our type exception declare const TypeException: unique symbol; // Basic type definitions type Struct = Record<string any>; type Funct<t r> = (arg: T) => R; type Types<t> = keyof T & string; type Sanitize<t> = T extends string ? T : never; // The core pattern for type-level exceptions export type Unbox<t extends struct> = { [Type in Types<t>]: T[Type] extends Funct<any infer ret> ? (arg: Ret) => any : T[Type] extends Struct ? { [TypeException]: `Variant }> is of type <union>. Migrate logic to <none> variant to capture }> types.`; } : (value: T[Type]) => any; }; </none></union></any></t></t></t></t></t></string>
How It Works
- TypeException is a unique symbol that acts as a special key for our error messages
- When we encounter an invalid type state, we return an object type with a TypeException property
- This type is unconstructable at runtime, forcing TypeScript to show our custom error message
- The error message can include type information using template literals
Example 1: Variant Handling with Custom Errors
Here's an example showing how to use this pattern with variant types:
type DataVariant = | { type: 'text'; content: string } | { type: 'number'; value: number } | { type: 'complex'; nested: { data: string } }; type VariantHandler = Unbox void; number: (value: number) => void; complex: { // This will trigger our custom error [TypeException]: `Variant <complex> is of type <union>. Migrate logic to <none> variant to capture <complex> types.` }; }>; // This will show our custom error at compile time const invalidHandler: VariantHandler = { text: (content) => console.log(content), number: (value) => console.log(value), complex: (nested) => console.log(nested) // Error: Type has unconstructable signature }; </complex></none></union></complex>
Example 2: Recursive Type Validation
Here's a more complex example showing how to use the pattern with recursive types:
type TreeNode<t> = { value: T; children?: TreeNode<t>[]; }; type TreeHandler<t> = Unbox void; node: TreeNode<t> extends Struct ? { [TypeException]: `Cannot directly handle node type. Use leaf handler for individual values.`; } : never; }>; // Usage example - will show custom error const invalidTreeHandler: TreeHandler<string> = { leaf: (value) => console.log(value), node: (node) => console.log(node) // Error: Cannot directly handle node type }; </string></t></t></t></t>
Example 3: Type State Validation
Here's how we can use the pattern to enforce valid type state transitions:
type LoadingState<t> = { idle: null; loading: null; error: Error; success: T; }; type StateHandler<t> = Unbox void; loading: () => void; error: (error: Error) => void; success: (data: T) => void; // Prevent direct access to state object state: LoadingState<t> extends Struct ? { [TypeException]: `Cannot access state directly. Use individual handlers for each state.`; } : never; }>; // This will trigger our custom error const invalidStateHandler: StateHandler<string> = { idle: () => {}, loading: () => {}, error: (e) => console.error(e), success: (data) => console.log(data), state: (state) => {} // Error: Cannot access state directly }; </string></t></t></t>
When to Use This Pattern
This pattern is particularly useful when:
- You need to prevent certain type combinations at compile time
- You want to provide clear, descriptive error messages for type violations
- You're building complex type hierarchies where certain operations should be restricted
- You need to guide developers toward correct usage patterns with helpful error messages
Technical Details
Let's break down how the pattern works internally:
// Create a unique symbol for our type exception declare const TypeException: unique symbol; // Basic type definitions type Struct = Record<string any>; type Funct<t r> = (arg: T) => R; type Types<t> = keyof T & string; type Sanitize<t> = T extends string ? T : never; // The core pattern for type-level exceptions export type Unbox<t extends struct> = { [Type in Types<t>]: T[Type] extends Funct<any infer ret> ? (arg: Ret) => any : T[Type] extends Struct ? { [TypeException]: `Variant }> is of type <union>. Migrate logic to <none> variant to capture }> types.`; } : (value: T[Type]) => any; }; </none></union></any></t></t></t></t></t></string>
Benefits Over Traditional Approaches
- Clear Error Messages: Instead of TypeScript's default type errors, you get custom messages that explain exactly what went wrong
- Compile-Time Safety: All errors are caught during development, not at runtime
- Self-Documenting: Error messages can include instructions on how to fix the issue
- Type-Safe: Maintains full type safety while providing better developer experience
- Zero Runtime Cost: All checking happens at compile time with no runtime overhead
Conclusion
Using unconstructable types with custom error messages is a powerful pattern for creating self-documenting type constraints. It leverages TypeScript's type system to provide clear guidance at compile time, helping developers catch and fix issues before they become runtime problems.
This pattern is particularly valuable when building complex type systems where certain combinations should be invalid. By making invalid states unrepresentable and providing clear error messages, we can create more maintainable and developer-friendly TypeScript code.
The above is the detailed content of Rich Compile-Time Exceptions in TypeScript Using Unconstructable Types. For more information, please follow other related articles on the PHP Chinese website!
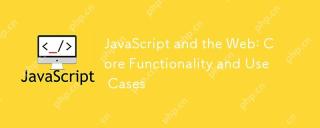
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
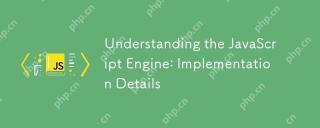
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
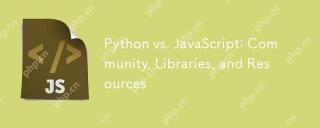
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
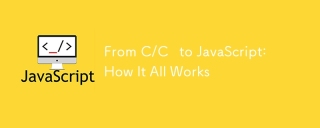
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
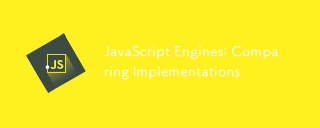
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
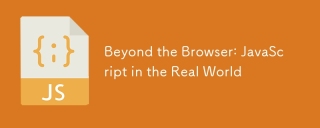
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
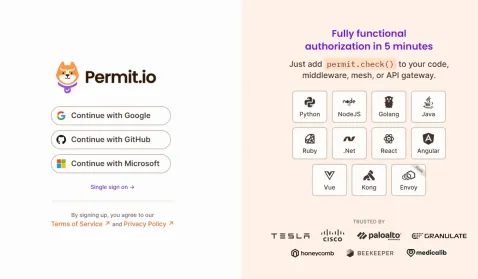
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
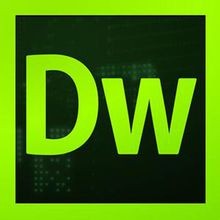
Dreamweaver CS6
Visual web development tools