


Java - Unable to Use ResultSet After Connection Closed
Problem:
An attempt to execute a database query using JDBC results in the following error:
java.sql.SQLException: Operation not allowed after ResultSet closed
Analysis:
JDBC utilizes a ResultSet object to retrieve data from a query. However, when the connection to the database is closed, the ResultSet object becomes invalid and can no longer be used.
Solution:
To resolve this issue, avoid passing the ResultSet object beyond the method that executed the query. Instead, use the ResultSet to populate an object or list and return that resulting object.
Example Code with Populating Objects:
<code class="java">public static Collection<t> sqlquery (String query, RowMapper<t> rowMapper) throws SQLException { Connection connection=null; Statement st=null; ResultSet rs=null; connection = DriverManager.getConnection("databaseadress","username","password"); st = connection.createStatement(); rs = st.executeQuery(query); Collection<t> collection = new ArrayList(); while (rs.next()) { collection.add(rowMapper.mapRow(rs)); } // Close resources even if exceptions are thrown closeGracefully(rs, st, connection); return collection; }</t></t></t></code>
Closing Resources Gracefully:
<code class="java">private static void closeGracefully(ResultSet rs, Statement st, Connection connection) { if (rs != null) { try { rs.close(); } catch (SQLException e) { /* Log or ignore */ } } if (st != null) { try { st.close(); } catch (SQLException e) { /* Log or ignore */ } } if (connection != null) { try { connection.close(); } catch (SQLException e) { /* Log or ignore */ } } }</code>
Additional Considerations:
- Use a row mapper to create objects from each row of the ResultSet.
- Parameterize queries to prevent SQL injection attacks.
- Consider utilizing third-party libraries such as JDBC or Spring JDBC for simplified database access.
The above is the detailed content of Why Am I Getting \'java.sql.SQLException: Operation not allowed after ResultSet closed\' When Using JDBC?. For more information, please follow other related articles on the PHP Chinese website!

MySQL processes data replication through three modes: asynchronous, semi-synchronous and group replication. 1) Asynchronous replication performance is high but data may be lost. 2) Semi-synchronous replication improves data security but increases latency. 3) Group replication supports multi-master replication and failover, suitable for high availability requirements.
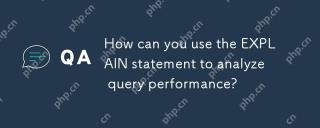
The EXPLAIN statement can be used to analyze and improve SQL query performance. 1. Execute the EXPLAIN statement to view the query plan. 2. Analyze the output results, pay attention to access type, index usage and JOIN order. 3. Create or adjust indexes based on the analysis results, optimize JOIN operations, and avoid full table scanning to improve query efficiency.
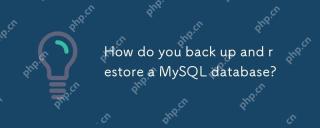
Using mysqldump for logical backup and MySQLEnterpriseBackup for hot backup are effective ways to back up MySQL databases. 1. Use mysqldump to back up the database: mysqldump-uroot-pmydatabase>mydatabase_backup.sql. 2. Use MySQLEnterpriseBackup for hot backup: mysqlbackup--user=root-password=password--backup-dir=/path/to/backupbackup. When recovering, use the corresponding life
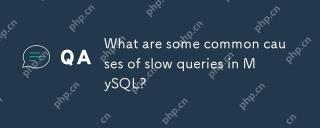
The main reasons for slow MySQL query include missing or improper use of indexes, query complexity, excessive data volume and insufficient hardware resources. Optimization suggestions include: 1. Create appropriate indexes; 2. Optimize query statements; 3. Use table partitioning technology; 4. Appropriately upgrade hardware.
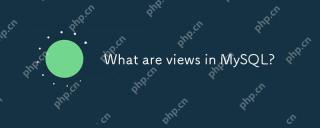
MySQL view is a virtual table based on SQL query results and does not store data. 1) Views simplify complex queries, 2) Enhance data security, and 3) Maintain data consistency. Views are stored queries in databases that can be used like tables, but data is generated dynamically.
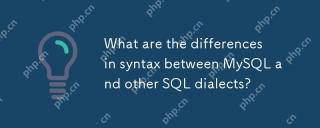
MySQLdiffersfromotherSQLdialectsinsyntaxforLIMIT,auto-increment,stringcomparison,subqueries,andperformanceanalysis.1)MySQLusesLIMIT,whileSQLServerusesTOPandOracleusesROWNUM.2)MySQL'sAUTO_INCREMENTcontrastswithPostgreSQL'sSERIALandOracle'ssequenceandt
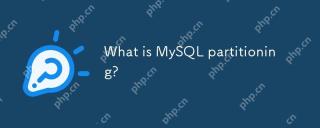
MySQL partitioning improves performance and simplifies maintenance. 1) Divide large tables into small pieces by specific criteria (such as date ranges), 2) physically divide data into independent files, 3) MySQL can focus on related partitions when querying, 4) Query optimizer can skip unrelated partitions, 5) Choosing the right partition strategy and maintaining it regularly is key.
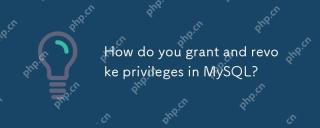
How to grant and revoke permissions in MySQL? 1. Use the GRANT statement to grant permissions, such as GRANTALLPRIVILEGESONdatabase_name.TO'username'@'host'; 2. Use the REVOKE statement to revoke permissions, such as REVOKEALLPRIVILEGESONdatabase_name.FROM'username'@'host' to ensure timely communication of permission changes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
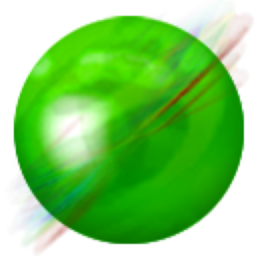
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use
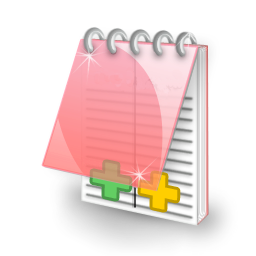
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
