


How to set a background picture in JPanel
Introduction
Setting a background image for a JPanel is relatively straightforward and can be accomplished in a variety of ways. Two common approaches are:
Approach 1: Using a JLabel
This approach involves creating a JLabel, setting its icon property to the desired image, and adding it to the JPanel as its content pane. However, this solution has the potential for the content to spill over if the required space for child components exceeds the size of the background image.
Approach 2: Creating a Custom Component
This approach involves extending a component like JPanel and overriding the paintComponent method to draw the background image in a custom way. This provides more flexibility in controlling how the image is scaled based on available space.
Additional Considerations
When setting a background image, it's important to ensure that the image is loaded correctly through APIs like ImageIO and use the appropriate location path based on whether the image is external or embedded within the application.
Example Using Approach 2:
The following code example demonstrates how to create a custom component to set a background image for a JPanel:
<code class="java">import java.awt.Dimension; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Image; import java.awt.RenderingHints; import java.awt.event.ComponentAdapter; import java.awt.event.ComponentEvent; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import javax.swing.JPanel; public class BackgroundPane extends JPanel { private BufferedImage img; private BufferedImage scaled; public BackgroundPane() { addComponentListener(new ComponentAdapter() { @Override public void componentResized(ComponentEvent e) { if (img != null) { Dimension size = BackgroundPane.this.getSize(); if (getWidth() > img.getWidth() || getHeight() > img.getHeight()) { scaled = getScaledInstanceToFill(img, size); } else { scaled = img; } } } }); } public void setBackground(BufferedImage value) { if (value != img) { this.img = value; scaled = img; repaint(); } } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); if (scaled != null) { int x = (getWidth() - scaled.getWidth()) / 2; int y = (getHeight() - scaled.getHeight()) / 2; g.drawImage(scaled, x, y, this); } } public static BufferedImage getScaledInstanceToFill(BufferedImage img, Dimension size) { double scaleFactor = getScaleFactorToFill(img, size); return getScaledInstance(img, scaleFactor); } public static double getScaleFactorToFill(BufferedImage img, Dimension size) { double dScale = 1; if (img != null) { int imageWidth = img.getWidth(); int imageHeight = img.getHeight(); double dScaleWidth = getScaleFactor(imageWidth, size.width); double dScaleHeight = getScaleFactor(imageHeight, size.height); dScale = Math.max(dScaleHeight, dScaleWidth); } return dScale; } public static double getScaleFactor(int iMasterSize, int iTargetSize) { double dScale = (double) iTargetSize / (double) iMasterSize; return dScale; } public static BufferedImage getScaledInstance(BufferedImage img, double dScaleFactor) { return getScaledInstance(img, dScaleFactor, RenderingHints.VALUE_INTERPOLATION_BILINEAR, true); } public static BufferedImage getScaledInstance(BufferedImage img, double dScaleFactor, int hint, boolean bHighQuality) { int width = (int) Math.round(img.getWidth() * dScaleFactor); int height = (int) Math.round(img.getHeight() * dScaleFactor); int type = (img.getTransparency() == Transparency.OPAQUE) ? BufferedImage.TYPE_INT_RGB : BufferedImage.TYPE_INT_ARGB; BufferedImage ret = (BufferedImage) img; if (width != img.getWidth() || height != img.getHeight()) { ret = new BufferedImage(width, height, type); Graphics2D g2 = ret.createGraphics(); g2.setRenderingHint(RenderingHints.KEY_INTERPOLATION, hint); g2.drawImage(img, 0, 0, width, height, null); g2.dispose(); } return ret; } }</code>
To use this custom component, simply instantiate it, set the background image using the setBackground method, and add the component to your JPanel as follows:
<code class="java">JPanel panel = new JPanel(); BackgroundPane background = new BackgroundPane(); background.setBackground(ImageIO.read(new File("/path/to/image.jpg"))); panel.add(background);</code>
This approach allows you to have greater control over the scaling of the background image based on the available space.
The above is the detailed content of How do you set a background image for a JPanel in Java, ensuring the image scales appropriately to the panel\'s size?. For more information, please follow other related articles on the PHP Chinese website!
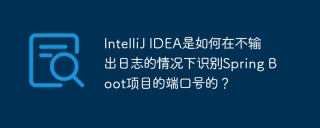
Start Spring using IntelliJIDEAUltimate version...
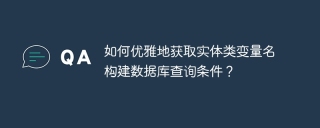
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
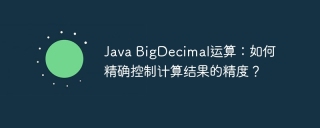
Java...
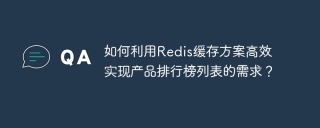
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
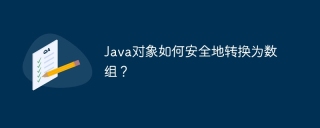
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
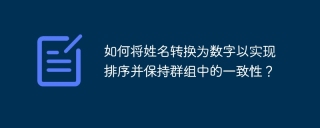
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
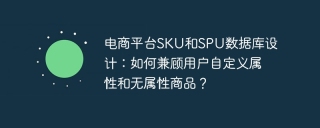
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
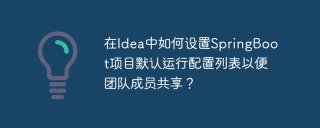
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
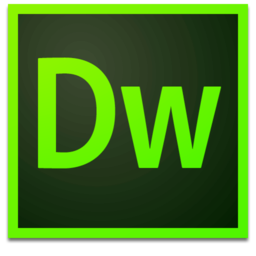
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
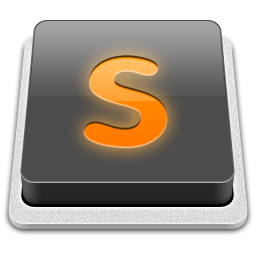
SublimeText3 Mac version
God-level code editing software (SublimeText3)
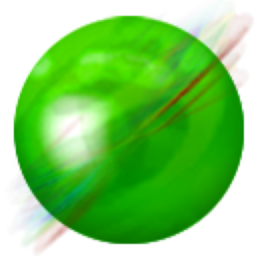
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment