Simulating TCP Connections in Go for Enhanced Network Testing
For thorough network testing, simulating TCP connections is crucial. In Go, a TCP connection is represented as an io.ReadWriteCloser, but customizing one for testing can be complex. Let's explore a solution that allows you to:
- Store data to be read in a string.
- Capture and access written data in a buffer.
Solution: Leveraging net.Pipe()
Enter net.Pipe(), a powerful function in the Go standard library. It creates two net.Conn instances:
- reader: a stream from which you can read the simulated data.
- writer: a stream to which you can write data that will be captured in the buffer.
Implementation:
<code class="go">package main import ( "fmt" "io" "net" ) func main() { // Create the simulated TCP connection reader, writer := net.Pipe() // Write data to the simulated connection _, err := io.WriteString(writer, "Hello, world!") if err != nil { panic(err) } // Read the data from the simulated connection buf := make([]byte, 1024) n, err := reader.Read(buf) if err != nil { panic(err) } fmt.Println(string(buf[:n])) // Prints "Hello, world!" }</code>
Benefits:
This approach offers several advantages:
- Isolation: The simulated connection is completely isolated from the actual network, allowing for controlled testing.
- Flexibility: You can easily modify the data to be read or written to test different scenarios.
- Extensibility: You can extend the solution to support additional features, such as mocking delays or errors.
By leveraging net.Pipe(), you can create simulated TCP connections in Go that fully satisfy your testing requirements, enabling you to thoroughly validate your network code.
The above is the detailed content of How can I Simulate TCP Connections in Go for Robust Network Testing?. For more information, please follow other related articles on the PHP Chinese website!
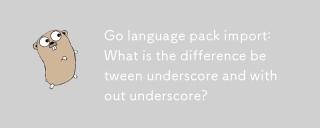
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
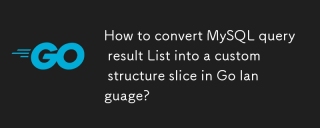
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
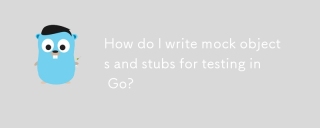
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
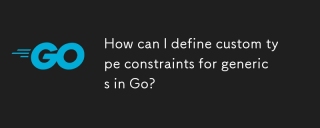
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
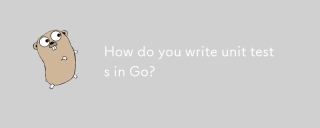
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
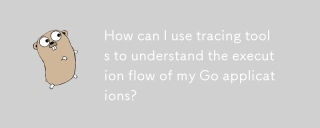
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
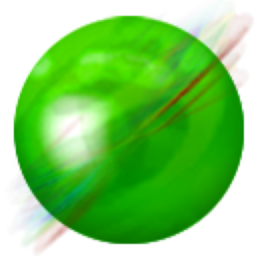
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
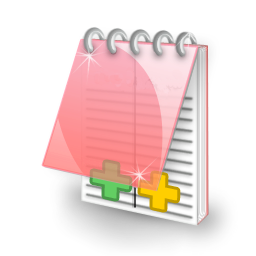
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
