


Custom Data Type Sharing in Go Plugins
In Go, you can define custom data types in plugins. However, sharing these data types between a plugin and the main application requires careful consideration.
The Limitations of Symbol Lookup
When accessing exported symbols from a plugin, you can type assert them into an interface. However, directly type asserting them into a struct is not possible using symbol lookup alone.
A Custom Data Type as an Example
Let's consider the following example:
<code class="go">// plugin.go package main type Person struct { Name string } var P Person = Person{ Name: "Emma", }</code>
<code class="go">// app.go package main import ( "fmt" "os" "plugin" ) func main() { plug, err := plugin.Open("./plugin.so") if err != nil { fmt.Println(err) os.Exit(1) } sym, err := plug.Lookup("P") if err != nil { fmt.Println(err) os.Exit(1) } var p Person p, ok := sym.(Person) if !ok { fmt.Println("Wrong symbol type") os.Exit(1) } fmt.Println(p.Name) // Panic: "Wrong symbol type" }</code>
In this example, the P symbol is a Person struct. However, the type assertion in the main app fails with an error.
The Solution: Separate Type Definition
The key to sharing custom data types between a plugin and the main application is to define the type separately. This way, both the plugin and the main app can refer to the same type definition.
Example with Separate Type Definition
<code class="go">// type.go package filter type Filter struct { Name string Age int }</code>
<code class="go">// plugin.go package main import "play/filter" var MyFilter filter.Filter = filter.Filter{ Name: "Bob", Age: 21, } func CreateFilter() filter.Filter { return filter.Filter{ Name: "Bob", Age: 21, } }</code>
<code class="go">// app.go package main import ( "fmt" "log" "os" "play/filter" "plugin" ) func main() { p, err := plugin.Open("plugin.so") if err != nil { log.Fatal(err) } mf, err := p.Lookup("MyFilter") if err != nil { log.Fatal(err) } f, ok := mf.(*filter.Filter) if !ok { log.Fatal("Wrong symbol type") } fmt.Printf("%+v\n", f) // Output: &{Name:Bob Age:21} }</code>
By defining the Filter type in a separate package, the plugin and the main app can both import it and use it for type assertions.
The above is the detailed content of How to Share Custom Data Types Between Go Plugins and the Main Application?. For more information, please follow other related articles on the PHP Chinese website!
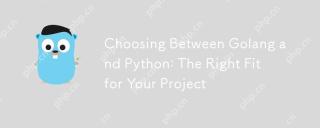
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
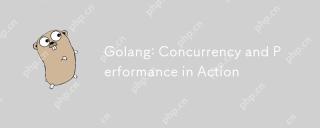
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
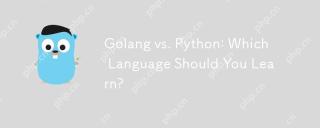
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
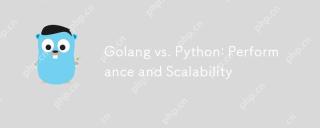
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
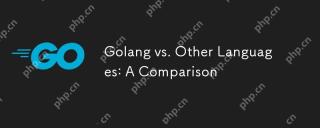
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
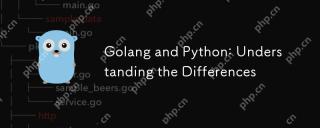
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
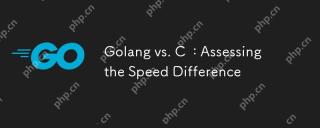
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
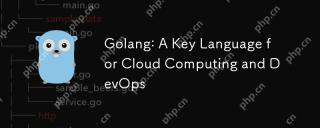
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
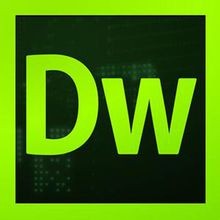
Dreamweaver CS6
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.