How to Synchronize Multithreaded Execution
In multithreaded applications, it's often necessary to coordinate the execution of parallel tasks. This article explores a method to wait for a group of threads to complete before proceeding.
The Problem:
Consider the following code:
<code class="java">public class DoSomethingInAThread implements Runnable {...} public static void main(String[] args) { for (int n=0; n<p>In this example, multiple new threads are created and started. However, the main() method continues execution immediately, without waiting for these threads to finish their tasks.</p> <p><strong>The Solution:</strong></p> <p>To synchronize the execution, we can create an array of thread references and then use the join() method on each thread object. This blocks the current thread until the target thread has completed its execution:</p> <pre class="brush:php;toolbar:false"><code class="java">import java.lang.Thread; public class DoSomethingInAThread implements Runnable {...} public static void main(String[] args) { Thread[] threads = new Thread[1000]; for (int n=0; n<threads.length n threads new thread dosomethinginathread for t: t.join><p>In this revised code, the main() method creates an array of thread references and starts each thread. It then enters a loop that calls join() on every thread in the array. This ensures that all threads complete their tasks before the main() method continues.</p></threads.length></code>
The above is the detailed content of How to Wait for Multiple Threads to Finish Execution Before Proceeding?. For more information, please follow other related articles on the PHP Chinese website!
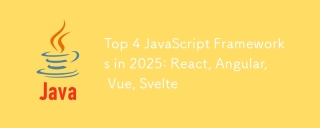
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
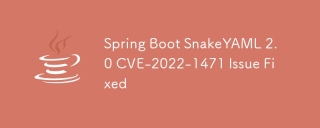
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
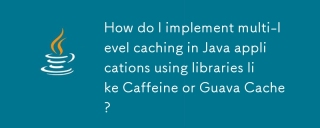
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
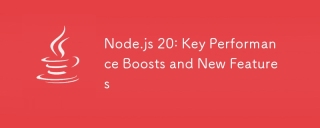
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
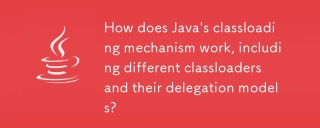
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
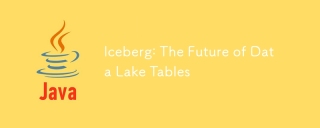
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
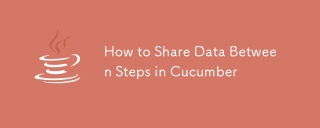
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
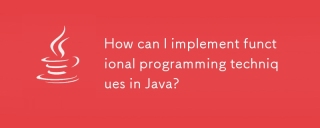
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
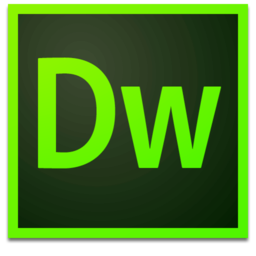
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
