


Indexing Interface Types in Go: Resolving the "Invalid Operation" Error
In Go, encountering the error "invalid operation: type interface {} does not support indexing" when attempting to index an interface type (interface{}) can be frustrating. This error occurs when you try to access a field or method of an interface without first asserting it to a specific data type.
Problem:
Consider the following code snippet:
<code class="go">var d interface{} json.NewDecoder(response.Body).Decode(&d) test := d["data"].(map[string]interface{})["type"]</code>
When trying to access the "type" field of the nested JSON response stored in d, you may encounter the "invalid operation" error. This is because d is of type interface{}, which abstracts over any type and does not inherently support indexing.
Solution:
To resolve this error, you need to perform type assertions to convert the interface to a known type that supports indexing. In this case, since you expect d to be a map, you can use the following code:
<code class="go">test := d.(map[string]interface{})["data"].(map[string]interface{})["type"] fmt.Println(test)</code>
Firstly, you assert d to be a map[string]interface{}. This allows you to index it by string keys. Then, you access the "data" field and repeat the assertion process to obtain its value.
Alternative Approach:
Alternatively, you can declare d to be map[string]interface{} directly, eliminating the need for the first type assertion:
<code class="go">var d map[string]interface{} if err := json.NewDecoder(response.Body).Decode(&d); err != nil { panic(err) } test := d["data"].(map[string]interface{})["type"] fmt.Println(test)</code>
This code retains the same functionality as the previous approach.
Additional Note:
If you encounter this indexing issue frequently, consider utilizing a library like github.com/icza/dyno, which simplifies working with dynamic objects in Go.
The above is the detailed content of How to Resolve the \'Invalid Operation: Type Interface {} Does Not Support Indexing\' Error in Go?. For more information, please follow other related articles on the PHP Chinese website!
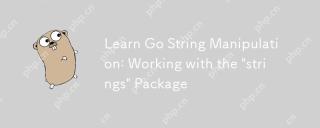
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
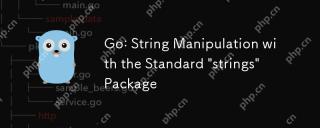
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
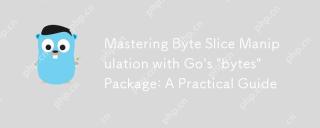
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
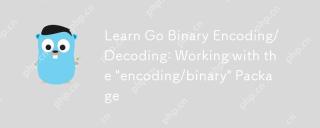
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
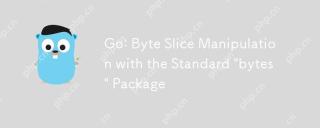
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
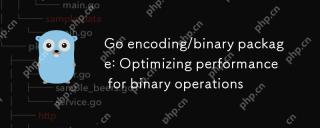
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
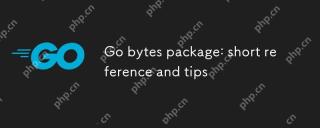
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
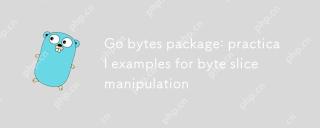
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
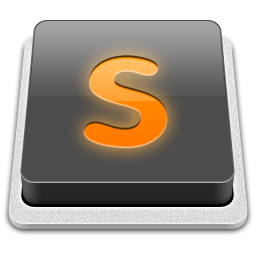
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment
