


Utilizing Nested Structs in GAE Datastore with Go
The Google App Engine datastore doesn't inherently support nested structs. However, there are techniques for achieving this functionality effectively.
One approach is to embed the user information within the post struct. For example, consider the following struct definitions:
<code class="go">type Post struct { Field1 string Field2 string User User // Nested user struct } type User struct { UserField1 string UserField2 string }</code>
By utilizing Go's PropertyLoadSaver interface, you can customize how your structs are serialized and deserialized from the datastore. This allows you to control how the user information is stored and retrieved.
<code class="go">// Implement PropertyLoadSaver interface to serialize/deserialize nested struct func (u *User) Load(props []datastore.Property) error { for _, prop := range props { switch prop.Name { case "UserField1": u.UserField1 = prop.Value.(string) case "UserField2": u.UserField2 = prop.Value.(string) } } return nil } func (u *User) Save() ([]datastore.Property, error) { props := []datastore.Property{ datastore.StringProperty("UserField1", u.UserField1), datastore.StringProperty("UserField2", u.UserField2), } return props, nil }</code>
By implementing this interface, you can ensure that the user information is stored as nested properties within the Post entity. This structure allows you to query and retrieve the nested user information along with the post data efficiently.
<code class="go">// Fetch the post and its embedded user information key := datastore.NameKey("Post", "my-post", nil) post := &Post{} if err := datastore.Get(ctx, key, post); err != nil { // Handle error } // JSON Marshal the post with its embedded user information jsonPost, err := json.Marshal(post) if err != nil { // Handle error }</code>
This approach provides a flexible and efficient solution for working with nested structs in the GAE datastore using Go.
The above is the detailed content of How Can I Effectively Implement Nested Structs in Google App Engine Datastore with Go?. For more information, please follow other related articles on the PHP Chinese website!
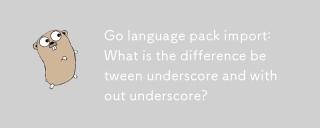
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
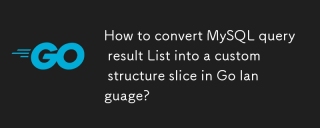
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
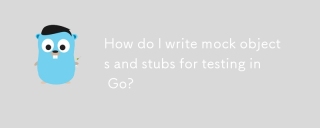
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
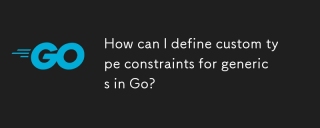
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
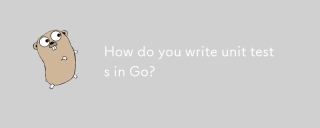
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
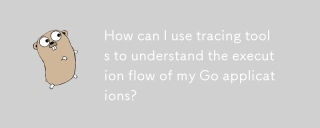
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
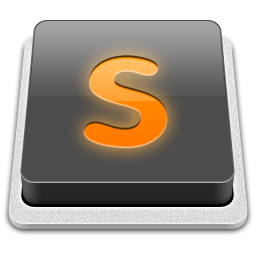
SublimeText3 Mac version
God-level code editing software (SublimeText3)
