


Implementing a Grid-Based Pixel Editor in Java
In the pursuit of enhancing编程 programming proficiency, developers often embark on creating fundamental applications such as pixel editors. A pixel editor's core functionality involves the user selecting colors and modifying the grid cells on the canvas, akin to popular image editors.
One question that frequently arises is the optimal choice of Java component for implementing such a grid-based system. While using JButtons as individual cells may appear intuitive, it can become inefficient and impractical, especially for larger grids.
Fortunately, a more efficient approach exists. By utilizing the drawImage() method and scaling the mouse coordinates, developers can create sizeable pixels.
To demonstrate this technique, consider the following example:
Grid.java
<code class="java">// Import required Java library import java.awt.Dimension; import java.awt.EventQueue; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Point; import java.awt.event.MouseEvent; import java.awt.event.MouseMotionListener; import java.awt.image.BufferedImage; import javax.swing.Icon; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.UIManager; /** * This class extends JPanel to create a grid-based pixel editor. * @see <a href="https://stackoverflow.com/questions/2900801">Original question</a> */ public class Grid extends JPanel implements MouseMotionListener { // Create buffered image for drawing private final BufferedImage img; // Image and panel dimensions private int imgW, imgH, paneW, paneH; public Grid(String name) { // Initialize basic attributes super(true); // Get the image icon and its dimensions Icon icon = UIManager.getIcon(name); imgW = icon.getIconWidth(); imgH = icon.getIconHeight(); // Set preferred size for the panel this.setPreferredSize(new Dimension(imgW * 10, imgH * 10)); // Create a BufferedImage for the image img = new BufferedImage(imgW, imgH, BufferedImage.TYPE_INT_ARGB); // Get Graphics2D object for drawing Graphics2D g2d = (Graphics2D) img.getGraphics(); // Draw the image icon on the BufferedImage icon.paintIcon(null, g2d, 0, 0); // Dispose the Graphics2D object g2d.dispose(); // Add MouseMotionListener to the panel this.addMouseMotionListener(this); } @Override protected void paintComponent(Graphics g) { // Get current panel dimensions paneW = this.getWidth(); paneH = this.getHeight(); // Draw the image on the panel with scaling g.drawImage(img, 0, 0, paneW, paneH, null); } @Override public void mouseMoved(MouseEvent e) { // Calculate mouse coordinates scaled to image size Point p = e.getPoint(); int x = p.x * imgW / paneW; int y = p.y * imgH / paneH; // Get the pixel color at the calculated scaled coordinates int c = img.getRGB(x, y); // Set tooltip text with color information this.setToolTipText(x + "," + y + ": " + String.format("%08X", c)); } @Override public void mouseDragged(MouseEvent e) { // Mouse drag functionality is not implemented in this example } // Helper method to create the GUI private static void create() { JFrame f = new JFrame(); f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); f.add(new Grid("Tree.closedIcon")); f.pack(); f.setVisible(true); } // Main method to run the application public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { @Override public void run() { create(); } }); } }</code>
By utilizing this technique, developers can effortlessly create pixel editors with large, scalable grids, enhancing the user experience while maintaining efficiency.
The above is the detailed content of How can I efficiently implement a grid-based pixel editor in Java, especially for large grids, without relying on JButtons for each cell?. For more information, please follow other related articles on the PHP Chinese website!
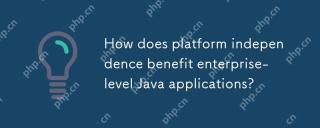
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
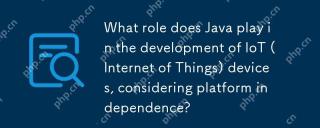
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
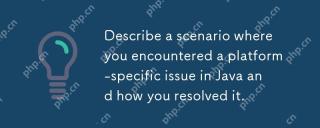
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
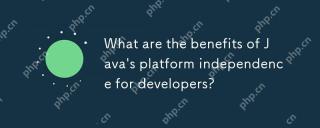
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
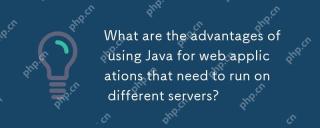
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
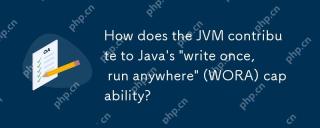
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
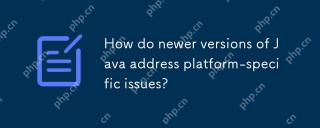
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
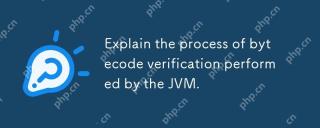
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
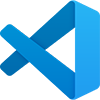
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
