


Why does the provided Go code with WaitGroup and buffered channel result in a deadlock?
Deadlocks in Go with WaitGroup and Buffered Channels
In Go, a deadlock occurs when multiple goroutines are waiting for each other to complete, resulting in a stalemate. This situation can arise when using buffered channels and WaitGroups incorrectly.
Consider the following code:
<code class="go">package main import "fmt" import "sync" func main() { ch := make(chan []int, 4) var m []int var wg sync.WaitGroup for i := 0; i <p>This code is expected to create a channel with a buffer size of 4 and start 5 goroutines, each sending an empty slice to the channel. The main goroutine waits for all goroutines to finish and then ranges over the channel.</p> <p>However, this code will result in a deadlock. Why?</p> <p><strong>Cause of the Deadlock:</strong></p> <p>Two problems exist in the code:</p> <ol> <li>Channel Capacity: The channel has a capacity of 4, which means it can hold up to 4 elements. However, there are 5 goroutines trying to send to the channel, resulting in a situation where the last goroutine will block waiting for a slot to be freed.</li> <li>Closing the Channel: The range ch loop continues to wait for elements to enter the channel. Since there are no more goroutines left to write to the channel, the loop will wait indefinitely.</li> </ol> <p><strong>Solutions:</strong></p> <ol> <li> <p><strong>Increase Channel Capacity:</strong> By increasing the channel capacity to 5, enough slots will be available for all goroutines to send their values without blocking. Additionally, closing the channel after the goroutines have finished writing will signal to the range loop that no more elements are coming, preventing it from waiting indefinitely.</p> <pre class="brush:php;toolbar:false"><code class="go">ch := make(chan []int, 5) ... wg.Wait() close(ch)</code>
Use Done() within the Loop: Instead of closing the channel, one can use the Done() method of WaitGroup to signal when the last goroutine has finished. By calling Done() within the range loop, the main goroutine will be notified when the channel is empty and the loop can exit.
<code class="go">go func() { for c := range ch { fmt.Printf("c is %v\n", c) wg.Done() } }() wg.Wait()</code>
These solutions resolve the deadlock by ensuring that the channel has sufficient capacity and that the range loop exits when there are no more elements to read from the channel.
The above is the detailed content of Why does the provided Go code with WaitGroup and buffered channel result in a deadlock?. For more information, please follow other related articles on the PHP Chinese website!
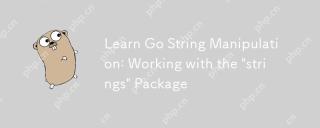
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
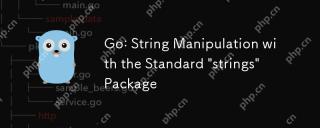
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
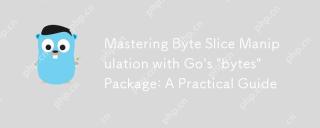
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
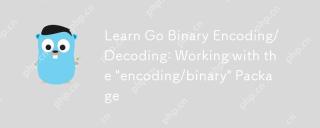
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
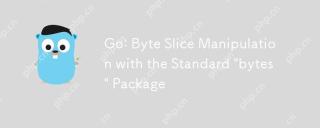
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
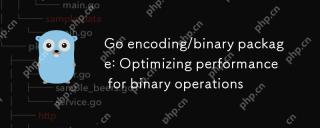
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
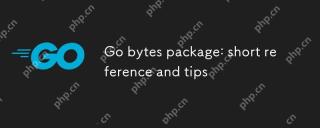
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
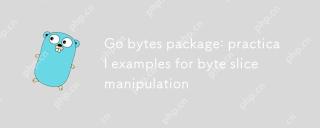
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
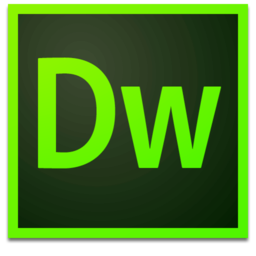
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
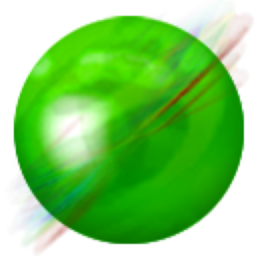
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
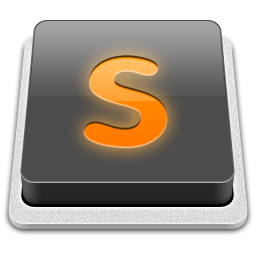
SublimeText3 Mac version
God-level code editing software (SublimeText3)
