JavaScript's Global Error Handling Mechanism: Catching Unhandled Errors
In JavaScript, it's essential to capture unhandled function errors. However, the language currently lacks a dedicated global error handling mechanism. This article delves into a solution to address this issue.
Assigning the window.onerror Event Handler
To capture all uncaught JavaScript errors, we can assign the window.onerror event to a custom event handler. The handler receives several parameters when an error occurs:
- msg: The error message
- url: The URL of the script that caused the error
- line: The line number within the script
- col (optional): The column number within the line (supported in HTML5)
- error (optional): The actual error object (supported in HTML5)
Code Example:
<code class="javascript">window.onerror = function(msg, url, line, col, error) { // Handle and log the error... };</code>
When Does window.onerror Fire?
The window.onerror event triggers whenever:
- An uncaught exception is thrown (e.g., throw "some message")
- A compile-time error occurs (e.g., missing closing bracket in a script)
Supported Browsers
The window.onerror event is supported in major browsers, including:
- Chrome 13
- Firefox 6.0
- Internet Explorer 5.5
- Opera 11.60
- Safari 5.1
Screenshot Example
AJAX Error Reporting
To facilitate error reporting, we can utilize AJAX to send the error details to a server-side script for logging or further analysis:
<code class="javascript">var xhr = new XMLHttpRequest(); xhr.open('POST', '/ajax/log_javascript_error'); xhr.setRequestHeader('X-Requested-With', 'XMLHttpRequest'); xhr.setRequestHeader('Content-Type', 'application/json'); xhr.onload = function() { ... }; xhr.send(JSON.stringify(errorData));</code>
References
For further exploration, refer to the following resources:
- Mozilla Developer Network :: window.onerror
- MSDN :: Handling and Avoiding Web Page Errors Part 2: Run-Time Errors
- Back to Basics – JavaScript onerror Event
- DEV.OPERA :: Better error handling with window.onerror
- Window onError Event
- Using the onerror event to suppress JavaScript errors
- SO :: window.onerror not firing in Firefox
The above is the detailed content of How Can You Implement Global Error Handling in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
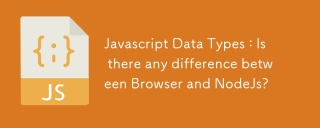
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
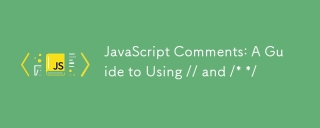
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
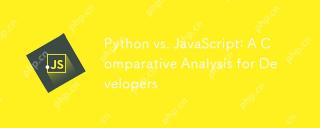
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
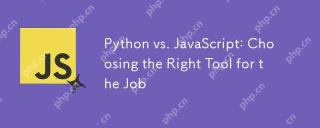
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
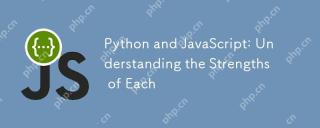
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
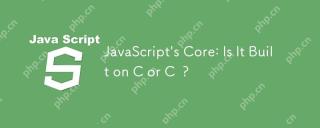
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
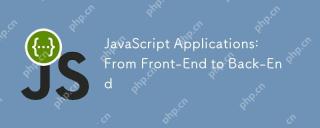
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
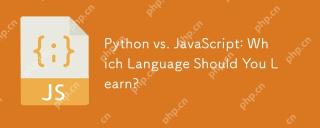
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
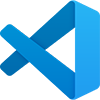
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
