


Why Binding Functions and Event Handlers Is Essential in React
Binding functions and event handlers in React ensures that when an event occurs, such as a button click or a form input change, the this context of the class method is properly bound. This is crucial because React components rely heavily on this to access their internal state and props.
When to Bind Functions in React
The need to bind functions arises when a class method needs to access the this context outside of the constructor or other class methods where the context is automatically bound. This is common when defining event handlers within the render method.
Different Binding Methods
In your example, you have three different ways of binding the someEventHandler method to the component:
<code class="js">// 1 return <input onchange="{this.someEventHandler.bind(this)}">; // 2 return <input onchange="{(event)"> this.someEventHandler(event)} />; // 3 return <input onchange="{this.someEventHandler}">;</code>
1. Binding with .bind(this)
This method creates a new function that explicitly binds the this context to the component. However, it's important to note that it creates a new function reference each time the component renders, which can be inefficient for performance-intensive operations.
2. Binding with a Function Arrow
This method uses an arrow function to define the event handler. In arrow functions, the this context is implicitly bound to the component. This also prevents the creation of a new function reference on each render, making it more efficient.
3. Binding Without Explicit Binding
This method simply passes the function as the callback without explicitly binding the this context. However, this approach requires that the function is bound in the constructor or elsewhere before it's called.
Choosing the Right Binding Method
The best binding method depends on the specific case and performance requirements:
- Pre-binding: Binding the function in the constructor or using arrow functions is recommended for performance-sensitive components.
- Runtime binding with .bind(this): Use this method for occasional event handlers or when you need to pass additional parameters.
- Runtime binding with arrow functions: Use this method for event handlers that need to access this but don't require additional parameters.
Final Thoughts
Binding functions and event handlers in React is essential to ensure proper functionality and performance. By understanding the different binding methods and their use cases, you can write optimized and maintainable code.
The above is the detailed content of Why and How to Bind Functions and Event Handlers in React: A Guide to Proper Function Binding for Optimal Performance and Functionality. For more information, please follow other related articles on the PHP Chinese website!
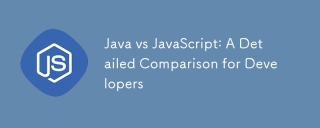
JavaandJavaScriptaredistinctlanguages:Javaisusedforenterpriseandmobileapps,whileJavaScriptisforinteractivewebpages.1)Javaiscompiled,staticallytyped,andrunsonJVM.2)JavaScriptisinterpreted,dynamicallytyped,andrunsinbrowsersorNode.js.3)JavausesOOPwithcl
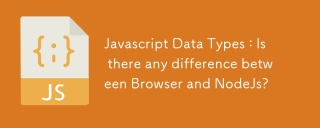
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
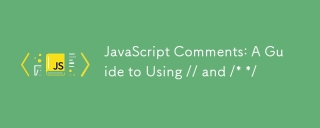
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
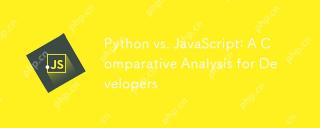
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
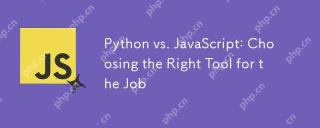
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
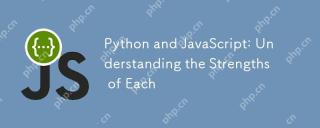
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
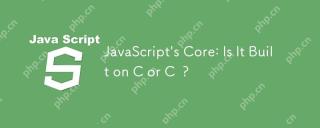
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
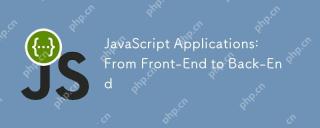
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
