


How can I efficiently insert data from Go structs into a PostgreSQL database using SQLX?
Inserting Struct Data into PostgreSQL with SQLX
Inserting data from a struct directly into a PostgreSQL database can be a time-consuming task when you have large structs with multiple fields. To streamline this process, consider using the SQLX library's NamedExec function.
Using SQLX for Struct Insertion
The SQLX library provides a simpler way to insert data from structs into PostgreSQL. Here's how you can use it:
-
Define DB Tags:
First, you need to define database field tags (db) for each struct field that you want to insert. This will help SQLX map the struct fields to database columns. -
Create a Named Query:
Construct a named query that includes the struct field tags as placeholders. For example:<code class="go">query := `INSERT INTO TABLENAME(leaveid, empid, supervisorid) VALUES(:leaveid, :empid, :supervisorid)`</code>
-
Execute the Query:
Use the NamedExec function to execute the query and pass in your struct as an argument. This will automatically populate the placeholders with the corresponding struct field values.<code class="go">var leave1 ApplyLeave1 _, err := db.NamedExec(query, leave1)</code>
Inserting JSON Arrays
To insert a JSON array into PostgreSQL, you can use the jsonb datatype. Define a []jsonb field in your struct and assign it a value of type []interface{}. For example:
<code class="go">type ApplyLeave1 struct { CertificateInfo []interface{} `db:"certificate"` }</code>
When inserting the struct, SQLX will automatically convert the []interface{} to a jsonb array in the database.
Additional Considerations:
-
Auto-Incrementing IDs:
If you want to use auto-incrementing IDs for your structs, you need to define a serial primary key field in the database and set its db tag to autoIncrement: true. -
Searching and Retrieval:
You can use indexes on your database columns to optimize search and retrieval operations. Additionally, SQLX provides more advanced query-building capabilities for complex operations. -
Updating Certificate Information:
To update specific certificate information, you can use queries like:<code class="go">query := `UPDATE TABLENAME SET certificatestatus = 'true' WHERE leaveid = ? AND certificateid = ?` _, err := db.Exec(query, leaveId, certificateId)</code>
Remember that your specific requirements may vary, so consult the SQLX documentation and PostgreSQL documentation for further guidance.
The above is the detailed content of How can I efficiently insert data from Go structs into a PostgreSQL database using SQLX?. For more information, please follow other related articles on the PHP Chinese website!
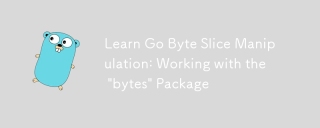
ThebytespackageinGoisessentialformanipulatingbytesliceseffectively.1)Usebytes.Jointoconcatenateslices.2)Employbytes.Bufferfordynamicdataconstruction.3)UtilizeIndexandContainsforsearching.4)ApplyReplaceandTrimformodifications.5)Usebytes.Splitforeffici
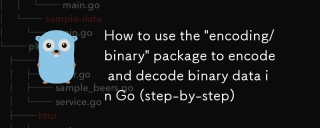
Tousethe"encoding/binary"packageinGoforencodinganddecodingbinarydata,followthesesteps:1)Importthepackageandcreateabuffer.2)Usebinary.Writetoencodedataintothebuffer,specifyingtheendianness.3)Usebinary.Readtodecodedatafromthebuffer,againspeci
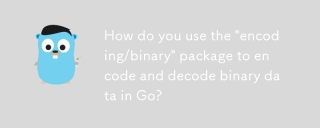
The encoding/binary package provides a unified way to process binary data. 1) Use binary.Write and binary.Read functions to encode and decode various data types such as integers and floating point numbers. 2) Custom types can be handled by implementing the binary.ByteOrder interface. 3) Pay attention to endianness selection, data alignment and error handling to ensure the correctness and efficiency of the data.

Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
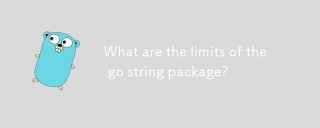
The strings package in Go has performance and memory usage limitations when handling large numbers of string operations. 1) Performance issues: For example, strings.Replace and strings.ReplaceAll are less efficient when dealing with large-scale string replacements. 2) Memory usage: Since the string is immutable, new objects will be generated every operation, resulting in an increase in memory consumption. 3) Unicode processing: It is not flexible enough when handling complex Unicode rules, and may require the help of other packages or libraries.
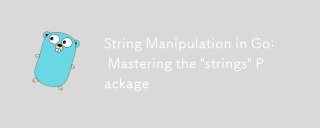
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
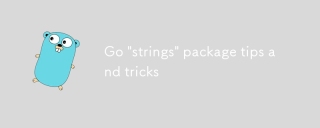
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
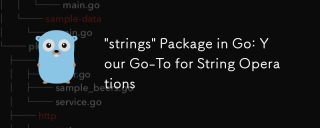
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
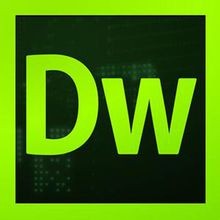
Dreamweaver CS6
Visual web development tools
