


How can I use a timer to display a modaless JDialog from another JFrame without using buttons?
Calling One JFrame from Another Using Timer Without Buttons
Instead of using buttons to trigger the display of a nested JFrame, consider employing a modeless dialog. This technique offers a cleaner and more efficient approach.
Implementation:
1. Modeless Dialog:
- Create a modeless JDialog, which is a top-level container that allows interaction with the underlying frame.
2. Timer for Countdown:
- Utilize a javax.swing.Timer to initiate a countdown that will automatically open the JDialog at a specific time interval.
3. PropertyChangeListener:
- Register a PropertyChangeListener for the JDialog to monitor changes in its value property.
4. Display Dialog:
- When a property change event is fired, hide the JDialog and dispatch a WINDOW_CLOSING event to close it.
Use Case:
The following code snippet demonstrates this technique:
<code class="java">import javax.swing.*; public class TimedDialogExample { // Countdown time in seconds private static final int TIME_OUT = 10; public static void main(String[] args) { SwingUtilities.invokeLater(() -> { // Create a frame JFrame frame = new JFrame("Main Frame"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(400, 300); // Create a dialog JDialog dialog = new JDialog(frame); dialog.setSize(300, 200); // Create a timer to display the dialog after TIME_OUT seconds Timer timer = new Timer(1000, e -> { // Hide the dialog dialog.setVisible(false); // Dispatch a WINDOW_CLOSING event to close the dialog dialog.dispatchEvent(new WindowEvent(dialog, WindowEvent.WINDOW_CLOSING)); }); timer.start(); // Set the dialog's content JPanel panel = new JPanel(); panel.add(new JLabel("This is the dialog")); dialog.add(panel); // Make the frame visible frame.setVisible(true); // Display the dialog after TIME_OUT seconds timer.stop(); dialog.setVisible(true); }); } }</code>
By employing this technique, you can automatically open a nested JDialog without the need for additional buttons. This approach provides a streamlined and intuitive user experience, especially when the timing of the display is crucial.
The above is the detailed content of How can I use a timer to display a modaless JDialog from another JFrame without using buttons?. For more information, please follow other related articles on the PHP Chinese website!
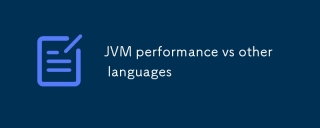
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
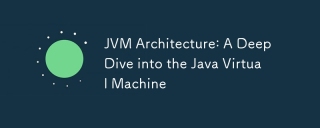
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
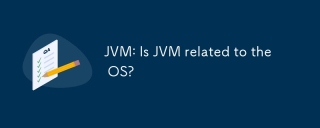
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
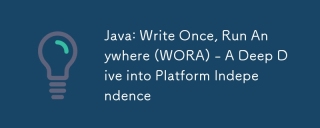
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
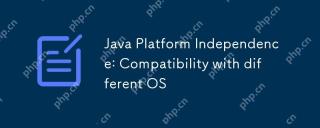
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
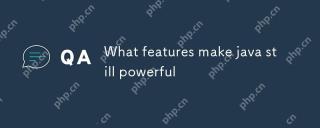
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
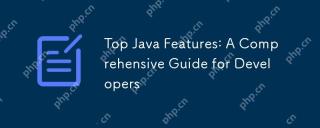
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
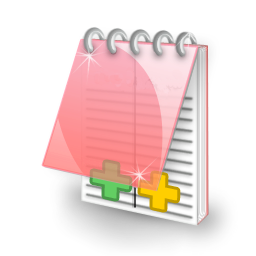
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
