Scrape multiple URLs with PyQt QWebPage
PyQt's QWebPage provides a means to render web pages, making it suitable for dynamically loaded content. However, attempting multiple renderings may result in crashes or unexpected behavior.
Problem Identification
The issue in the provided code stems from the creation of multiple QApplications and QWebPages for each URL fetch. Instead, a single instance of each should be utilized, with the WebPage relying on its loadFinished signal to trigger internal processing of subsequent URLs.
Solution
The following improvements address the problem:
- One QApplication and WebPage Instance: Create a single QApplication and WebPage, avoiding redundant instantiations.
- Internal Processing Loop: Utilize the loadFinished signal to fetch URLs sequentially, implementing an internal processing loop within the WebPage.
- Custom HTML Processing: Connect a user-defined slot to the htmlReady signal, which emits HTML and URL information after each page load.
Usage
Example code demonstrating how to use the improved WebPage:
def my_html_processor(html, url): print('loaded: [%d chars] %s' % (len(html), url)) import sys app = QApplication(sys.argv) webpage = WebPage(verbose=False) webpage.htmlReady.connect(my_html_processor) # example 1: process list of urls urls = ['https://en.wikipedia.org/wiki/Special:Random'] * 3 print('Processing list of urls...') webpage.process(urls) # example 2: process one url continuously import signal, itertools signal.signal(signal.SIGINT, signal.SIG_DFL) print('Processing url continuously...') print('Press Ctrl+C to quit') url = 'https://en.wikipedia.org/wiki/Special:Random' webpage.process(itertools.repeat(url)) sys.exit(app.exec_())
References
- [PyQt5 WebPage](https://doc.qt.io/qt-5/qwebenginepage.html)
- [PyQt4 WebPage](https://doc.qt.io/archives/qt-4.8/qwebpage.html)
The above is the detailed content of How to Efficiently Scrape Multiple URLs Using PyQt QWebPage?. For more information, please follow other related articles on the PHP Chinese website!
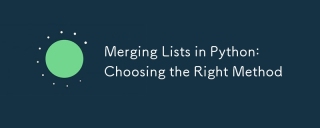
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
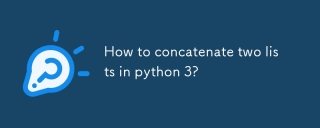
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
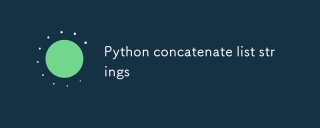
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
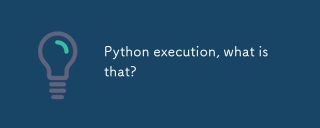
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
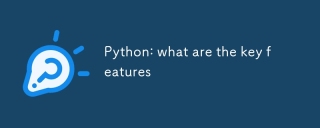
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
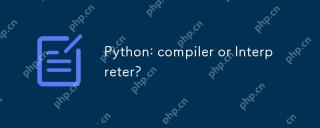
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
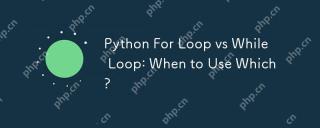
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
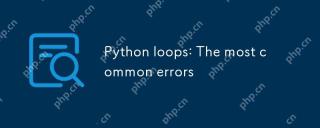
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
