


Ensuring Null Safety in Chains of Getter Calls
To safeguard against NullPointerExceptions in complex chains of getter calls, one common approach involves explicit null checks at each level, as exemplified in the code snippet provided.
However, this approach can become tedious and verbose, especially in scenarios with numerous getter calls. To address this, Java 8 introduced the Optional type, which elegantly manages null values and simplifies code structure.
Utilizing Optional to Handle Nulls
By utilizing Optional, you can seamlessly handle nulls in getter chains without violating the Law of Demeter. Optional enables you to chain multiple map operations, which effectively perform the getter calls. Null checks are handled automatically within this framework.
Consider the following example, where objects are not initialized:
<code class="java">System.out.println("----- Not Initialized! -----"); Optional.ofNullable(new Outer()) .map(out -> out.getNested()) .map(nest -> nest.getInner()) .map(in -> in.getFoo()) .ifPresent(foo -> System.out.println("foo: " + foo)); //no print</code>
In this case, no output will be generated since null values are encountered during the getter calls.
Now, let's initialize the objects:
<code class="java">System.out.println("----- Let's Initialize! -----"); Optional.ofNullable(new OuterInit()) .map(out -> out.getNestedInit()) .map(nest -> nest.getInnerInit()) .map(in -> in.getFoo()) .ifPresent(foo -> System.out.println("foo: " + foo)); //will print!</code>
This time, the program successfully prints "foo: yeah!", demonstrating how Optional handles null values gracefully.
Applying Optional to Your Getter Chain
For your specific getter chain involving house.getFloor(0).getWall(WEST).getDoor().getDoorknob(), you can utilize Optional in the following manner:
<code class="java">Optional.ofNullable(house) .map(house -> house.getFloor(0)) .map(floorZero -> floorZero.getWall(WEST)) .map(wallWest -> wallWest.getDoor()) .map(door -> wallWest.getDoor())</code>
This code snippet returns an Optional
The above is the detailed content of How can Optional in Java 8 help me avoid NullPointerExceptions in complex getter chains?. For more information, please follow other related articles on the PHP Chinese website!
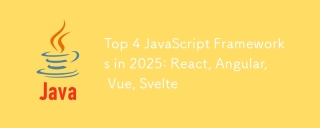
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
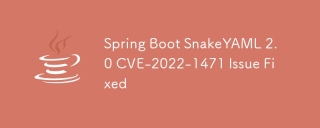
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
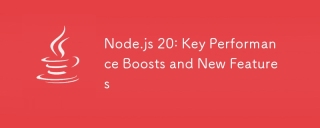
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
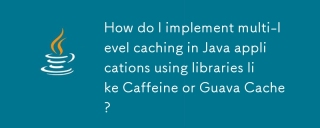
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
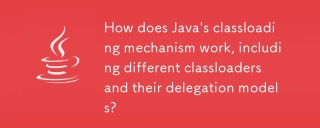
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
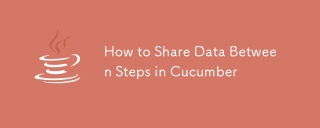
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
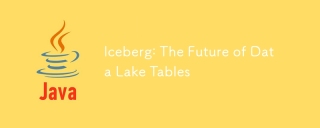
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
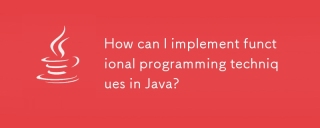
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
