


Laravel Authentication: Troubleshooting "make:auth Command Not Defined" Error
When attempting to run the make:auth command in Laravel 5.2, you may encounter an error message stating that the command is not defined. This issue can arise for various reasons, which we will explore in detail.
For Laravel versions 5.2 and older, the make:auth command is not available. The following commands are supported in Laravel 5.2:
- make:auth
- make:console
- make:controller
- make:entity
- make:event
- make:job
- make:listener
- make:middleware
- make:migration
- make:model
- make:policy
- make:presenter
- make:provider
- make:repository
- make:request
- make:seeder
- make:test
- make:transformer
If you are using Laravel 5.2, you can manually create the authentication views and routes by following these steps:
- Create the routes.php file in the routes directory.
-
Add the following code to the routes.php file:
<code class="php">// Authentication Routes... Route::get('login', 'Auth\LoginController@showLoginForm')->name('login'); Route::post('login', 'Auth\LoginController@login')->name('login.post'); Route::post('logout', 'Auth\LoginController@logout')->name('logout'); // Registration Routes... Route::get('register', 'Auth\RegisterController@showRegistrationForm')->name('register'); Route::post('register', 'Auth\RegisterController@register')->name('register.post'); // Password Reset Routes... Route::get('password/reset', 'Auth\ForgotPasswordController@showLinkRequestForm')->name('password.request'); Route::post('password/email', 'Auth\ForgotPasswordController@sendResetLinkEmail')->name('password.email'); Route::get('password/reset/{token}', 'Auth\ResetPasswordController@showResetForm')->name('password.reset'); Route::post('password/reset', 'Auth\ResetPasswordController@reset')->name('password.update');</code>
- Create the LoginController.php file in the app/Http/Controllers/Auth directory.
-
Add the following code to the LoginController.php file:
<code class="php">namespace App\Http\Controllers\Auth; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; use Illuminate\Support\Facades\Route; class LoginController extends Controller { public function showLoginForm() { return view('auth.login'); } public function login(Request $request) { $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials)) { // The user was authenticated return redirect()->intended(route('home')); } // The user could not be authenticated return redirect()->back()->withErrors(['email' => 'The provided credentials do not match our records.']); } public function logout(Request $request) { Auth::logout(); return redirect()->route('login'); } }</code>
- Create the RegisterController.php file in the app/Http/Controllers/Auth directory.
-
Add the following code to the RegisterController.php file:
<code class="php">namespace App\Http\Controllers\Auth; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; use Illuminate\Support\Facades\Route; class RegisterController extends Controller { public function showRegistrationForm() { return view('auth.register'); } public function register(Request $request) { $this->validate($request, [ 'name' => 'required|string|max:255', 'email' => 'required|string|email|max:255|unique:users', 'password' => 'required|string|min:6|confirmed', ]); // Create the user $user = User::create([ 'name' => $request->name, 'email' => $request->email, 'password' => bcrypt($request->password), ]); // Log the user in Auth::login($user); // Redirect the user to the home page return redirect()->route('home'); } }</code>
- Create the ForgotPasswordController.php file in the app/Http/Controllers/Auth directory.
-
Add the following code to the ForgotPasswordController.php file:
<code class="php">namespace App\Http\Controllers\Auth; use Illuminate\Http\Request; use Illuminate\Support\Facades\Password; class ForgotPasswordController extends Controller { public function showLinkRequestForm() { return view('auth.passwords.email'); } public function sendResetLinkEmail(Request $request) { $this->validate($request, [ 'email' => 'required|string|email|max:255', ]); // We will send the reset link to this user. Once we have attempted // to send the link, we will examine the response then see the message we // need to show to the user. Finally, we'll send out a proper // response. $response = Password::sendResetLink( $request->only('email') ); switch ($response) { case Password::RESET_LINK_SENT: return back()->with('status', __($response)); case Password::INVALID_USER: return back()->withErrors(['email' => __($response)]); } } }</code>
- Create the ResetPasswordController.php file in the app/Http/Controllers/Auth directory.
-
Add the following code to the ResetPasswordController.php file:
<code class="php">namespace App\Http\Controllers\Auth; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Password; use Illuminate\Validation\ValidationException; class ResetPasswordController extends Controller { public function showResetForm(Request $request, $token = null) { return view('auth.passwords.reset')->with( ['token' => $token, 'email' => $request->email] ); } public function reset(Request $request) { $request->validate([ 'token' => 'required', 'email' => 'required|email', 'password' => 'required|min:6|confirmed', ]); // Here we will attempt to reset the user's password. If it is successful we // will update the password on an existing user and return a response // indicating that the user's password has been reset. $response = Password::reset( $request->only('email', 'password', 'password_confirmation', 'token'), function ($user) use ($request) { $user->forceFill([ 'password' => Hash::make($request->password), 'remember_token' => Str::random(60), ])->save(); // In case of large user base, it's recommended to use // $user->setRememberToken(Str::random(60)); // $user->save(); } ); switch ($response) { case Password::PASSWORD_RESET: return redirect()->route('login')->with('status', __($response)); default: throw ValidationException::withMessages([ 'email' => [__($response)], ]); } } }</code>
- Open the resources/views/auth directory and create your views.
After completing these steps, you should have a working authentication system in your Laravel 5.2 application.
For Laravel >= 6
In Laravel 6 and above, the make:auth command has been replaced with the ui command. To create authentication views and routes using this command, run the following:
composer require laravel/ui php artisan ui vue --auth php artisan migrate
This command will install the Laravel UI package and create the necessary authentication views, routes, and migrations.
The above is the detailed content of Why is the \'make:auth\' command not defined in Laravel 5.2 and how can I manually set up authentication?. For more information, please follow other related articles on the PHP Chinese website!
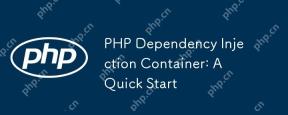
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
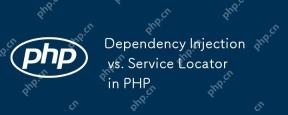
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
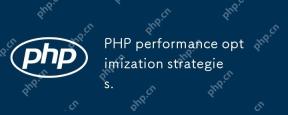
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
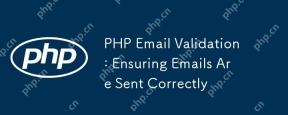
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
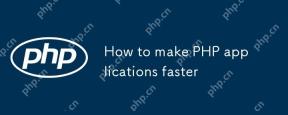
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
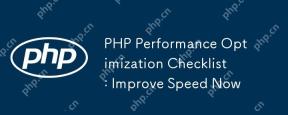
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
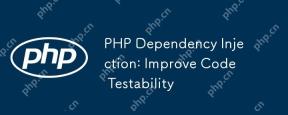
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
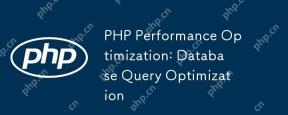
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
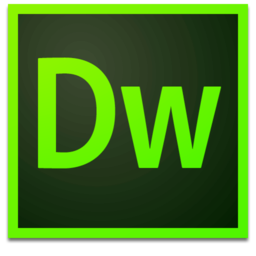
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
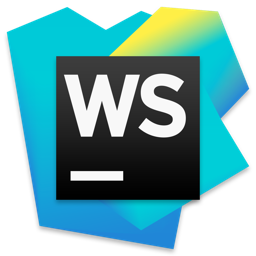
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
