Sorting Object Arrays with JQuery/JavaScript
In JavaScript, you may encounter arrays of objects that require sorting based on specific attributes. To sort an array of objects by a specific attribute, such as "name" in your case, follow these steps:
- Extract the Attributes: Create a helper function that extracts the desired attribute from each object in the array. In your case, it would be function(object) { return object.name; }.
- Define a Comparison Function: Implement a comparison function that takes two objects and compares their specified attributes. For sorting by "name" in ascending order, use function(a, b) { return a.name.toLowerCase() - b.name.toLowerCase(); }.
- Use Array.sort(): Apply the sort() method to the array with the provided comparison function as a parameter: array.sort(comparisonFunction);.
Example:
Consider the following array of objects:
<code class="javascript">var array = [ { id: 1, name: "Alice", value: 10 }, { id: 2, name: "Bob", value: 15 }, { id: 3, name: "Carl", value: 5 } ];</code>
To sort the array by "name" in ascending order, use the following function:
<code class="javascript">function SortByName(a, b) { var aName = a.name.toLowerCase(); var bName = b.name.toLowerCase(); return ((aName bName) ? 1 : 0)); }</code>
Apply the sorting using:
<code class="javascript">array.sort(SortByName);</code>
The resulting array will be sorted by "name" in ascending order:
<code class="javascript">[ { id: 3, name: "Carl", value: 5 }, { id: 1, name: "Alice", value: 10 }, { id: 2, name: "Bob", value: 15 } ]</code>
Regarding the Duplicate Question:
It appears that the question was previously closed as a duplicate because it was considered similar to a later question that received more attention. However, this question was asked earlier and should not have been marked as a duplicate.
The above is the detailed content of How to Sort Arrays of Objects by Attribute Using JQuery/JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
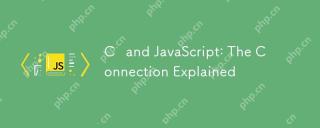
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
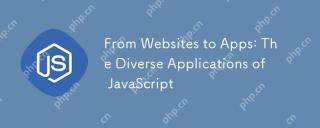
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
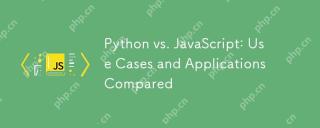
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
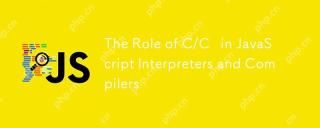
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
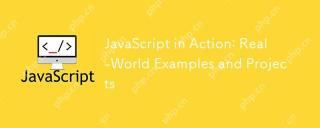
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
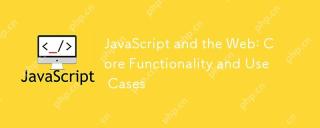
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
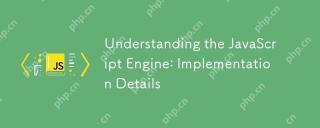
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
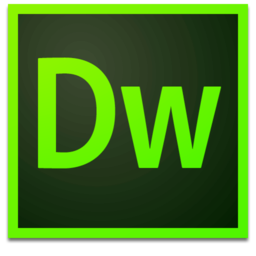
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
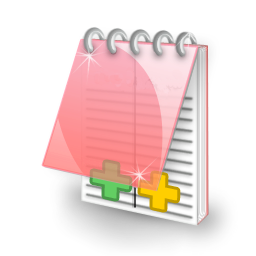
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function