


Peak-finding Algorithm for Python/SciPy
Finding peaks in data is a common task in signal processing and analysis. While it is possible to implement a peak-finding algorithm manually, it is often more convenient to use an existing library function.
One such function is scipy.signal.find_peaks. This function takes a signal as input and returns the indices of the peaks. It can be used for both 1D and 2D signals.
find_peaks has a number of parameters that control its behavior. These parameters include:
- distance: The minimum distance between peaks. This parameter ensures that only isolated peaks are returned.
- threshold: The minimum amplitude of a peak. This parameter ensures that only significant peaks are returned.
- width: The width of a peak. This parameter can be used to reject noise or to group multiple peaks into a single peak.
In addition to these parameters, find_peaks also has a number of advanced parameters, such as height and prominence. These parameters can be used to fine-tune the peak-finding algorithm for specific applications.
To use find_peaks, simply call the function with the signal as the first argument. The function will return a tuple containing the indices of the peaks and a dictionary containing the values of the advanced parameters.
Here is an example of how to use find_peaks to find peaks in a 1D signal:
<code class="python">import numpy as np from scipy.signal import find_peaks x = np.sin(2*np.pi*100*np.arange(1000)/1000) peaks, _ = find_peaks(x) plt.plot(x) plt.plot(peaks, x[peaks], "xr") plt.show()</code>
This code will plot the signal and the detected peaks. As you can see, the find_peaks function is able to accurately identify the peaks in the signal.
find_peaks is a versatile and powerful peak-finding algorithm that can be used for a wide range of applications. It is easy to use and provides a number of advanced parameters for fine-tuning the peak-finding process.
The above is the detailed content of How to Find Peaks in Data Using Python/SciPy\'s Peak-Finding Algorithm?. For more information, please follow other related articles on the PHP Chinese website!
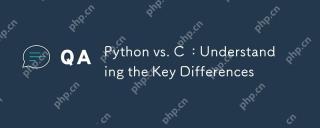
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
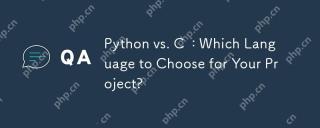
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
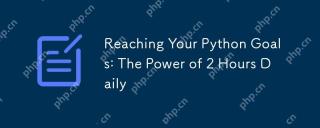
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
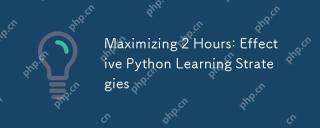
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
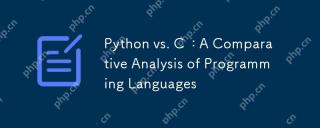
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
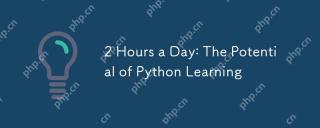
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
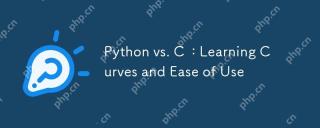
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
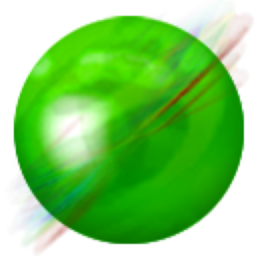
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
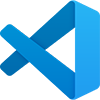
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment