Referencing Variables in Python
Introduction
In programming, referencing variables enables us to establish a connection between two variables, allowing changes made to one to be reflected in the other. While this concept is commonly achieved through references in languages like C , Python offers a different approach.
Reference Semantics in Python
Unlike C , Python does not have explicit references for variables. Instead, variables are assigned to objects, and these objects can be either mutable (changeable) or immutable (fixed). When assigning a variable to another variable, Python does not copy the object but rather creates a reference to the original object.
This means that any modification made to the object directly affects all variables referencing it. For example:
<code class="python">y = 7 x = y x = 8</code>
After this code, both y and x will have the value of 7. Changing x to 8 will not change y because Python considers them to be independent variables, each referring to its own copy of the value 7.
Simulating References Using Aliasing
Although Python does not support references in the same way as C , it is possible to simulate references by using mutable objects. For instance, one can create a custom class that behaves like a reference:
<code class="python">class Reference: def __init__(self, val): self._value = val def get(self): return self._value def set(self, val): self._value = val</code>
By using this class, one can create multiple variables referencing the same underlying value:
<code class="python">reference = Reference(7) x = reference y = reference</code>
Now, any changes made to x or y will be reflected in the underlying value referenced by reference, effectively simulating C -like references.
Conclusion
While Python does not have explicit references like C , its reference semantics for objects allow for the creation of simulated references using mutable objects. This enables the modification of variables to affect other variables referencing the same underlying value, providing a flexible way to establish connections between variables.
The above is the detailed content of How Does Python Handle References and Variable Relationships?. For more information, please follow other related articles on the PHP Chinese website!
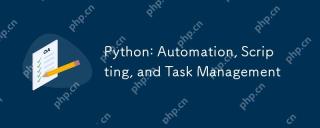
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
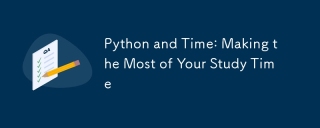
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
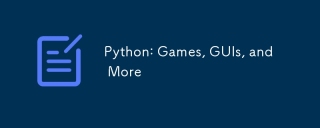
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
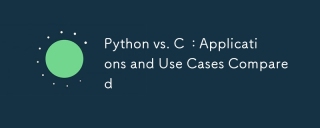
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
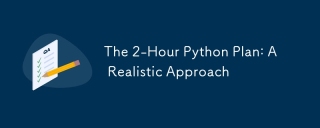
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
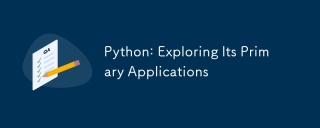
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
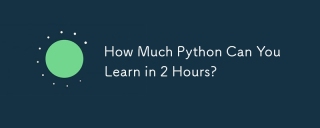
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
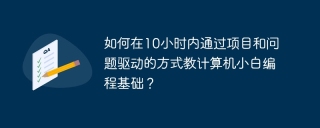
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
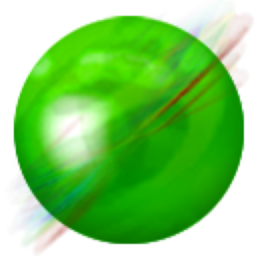
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor