


Understanding the "Cannot Read Property of Undefined" Error in React's map Function
In React development, it's common to encounter the error "TypeError: Cannot read property 'onPlayerScoreChange' of undefined" while utilizing the map function. This issue typically arises due to an incorrect binding process of the map function.
Context
In the provided code, we have a hierarchical component structure with the following components:
- Parent: App
- Child: Player
- Grandchild: Counter
The "onPlayerScoreChange" method is defined in the App component and is intended to update a player's score based on user input.
The Problem
The error occurs within the map function of the "App" component, where the "onScoreChange" prop is assigned to the "onPlayerScoreChange" method of the App component:
{this.state.initialPlayers.map(function(player, index) { return( <player name="{player.name}" score="{player.score}" key="{player.id}" index="{index}" onscorechange="{this.onPlayerScoreChange}"></player> ) })}
However, the context of the map function is distinct from the React component context. As a result, "this" inside the function refers to something other than the App component, making "this.onPlayerScoreChange" undefined.
The Solution
To resolve this issue, we need to bind the map function to the App component's context. This can be achieved either by using arrow (lambda) functions or the bind method.
Using Arrow Functions
{this.state.initialPlayers.map((player, index) => { return( <player name="{player.name}" score="{player.score}" key="{player.id}" index="{index}" onscorechange="{this.onPlayerScoreChange}"></player> ) })}
Arrow functions automatically bind the "this" value to the surrounding scope, eliminating the need for explicit binding.
Using the Bind Method
{this.state.initialPlayers.map(function(player, index) { return( <player name="{player.name}" score="{player.score}" key="{player.id}" index="{index}" onscorechange="{this.onPlayerScoreChange.bind(this)}"></player> ) }.bind(this))}
The bind method explicitly binds the map function to the App component's context.
The above is the detailed content of How to Resolve the \'Cannot Read Property of Undefined\' Error in React\'s map Function?. For more information, please follow other related articles on the PHP Chinese website!
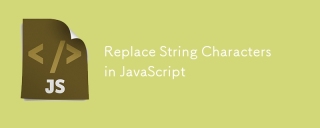
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
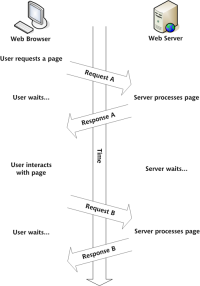
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
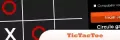
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
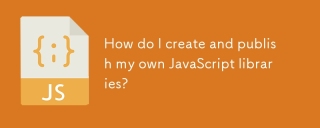
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
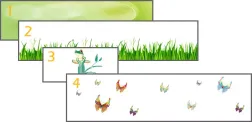
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
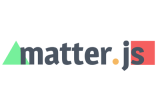
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
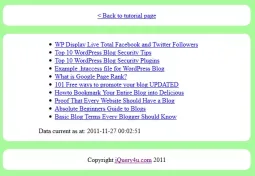
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
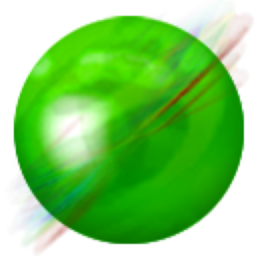
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
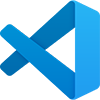
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.