


How to Selectively Remove Non-ASCII Characters Preserving Spaces and Periods?
Selective Removal of Non-ASCII Characters
Working with textual data often involves the need to remove non-ASCII characters, while preserving certain symbols like spaces and periods. While basic filtering methods may remove all non-ASCII characters, this might not be desirable in some cases.
Let's consider the following code:
<code class="python">def onlyascii(char): if ord(char) 127: return '' else: return char</code>
This code removes all characters with ASCII values less than 48 or greater than 127, effectively stripping the text of non-ASCII characters. However, it also removes spaces (ASCII 32) and periods (ASCII 46).
To selectively remove non-ASCII characters while preserving spaces and periods, we can leverage Python's string.printable module:
<code class="python">import string printable = set(string.printable) filtered_data = filter(lambda x: x in printable, data)</code>
The string.printable set contains all printable characters on the system, including digits, letters, symbols, spaces, and periods. Using this set as a filter, we can remove all non-printable characters from the string.
For example, if we have the string "somex00string. withx15 funny characters":
<code class="python">s = "some\x00string. with\x15 funny characters" ''.join(filter(lambda x: x in printable, s))</code>
The result will be:
'somestring. with funny characters'
This method effectively removes non-ASCII characters while preserving spaces and periods, providing a clean string for further processing.
The above is the detailed content of How to Selectively Remove Non-ASCII Characters Preserving Spaces and Periods?. For more information, please follow other related articles on the PHP Chinese website!
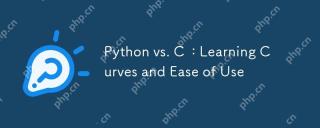
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
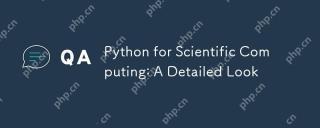
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
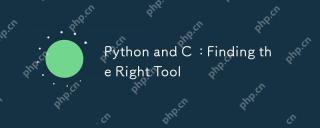
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
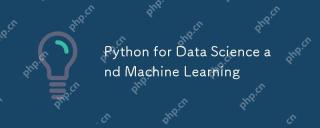
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
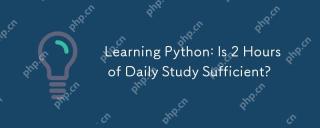
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
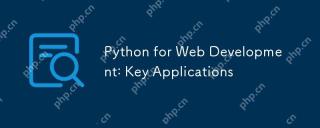
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
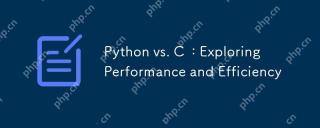
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
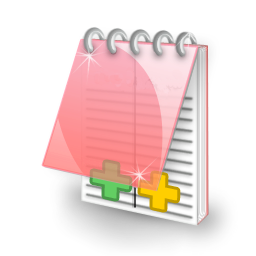
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
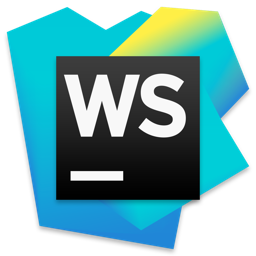
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.