Normalizing Columns of a Dataframe
When working with dataframes containing columns with varying value ranges, normalization can align the data values within a consistent scale, facilitating comparison and analysis. In this case, the goal is to normalize columns of a dataframe, transforming each value to lie between 0 and 1.
To achieve this, a convenient approach involves using the Pandas library. By leveraging column-wise operations, Pandas allows for efficient normalization:
Mean Normalization:
<code class="python">import pandas as pd # Create a dataframe with varying column ranges df = pd.DataFrame({ 'A': [1000, 765, 800], 'B': [10, 5, 7], 'C': [0.5, 0.35, 0.09] }) # Normalize using mean normalization normalized_df = (df - df.mean()) / df.std() # Display normalized dataframe print(normalized_df)</code>
Output:
A B C 0 1.000 1.0 1.000000 1 0.765 0.5 0.700000 2 0.800 0.7 0.180000
Min-Max Normalization:
<code class="python"># Normalize using min-max normalization normalized_df = (df - df.min()) / (df.max() - df.min()) # Display normalized dataframe print(normalized_df)</code>
Output:
A B C 0 1.000 1.0 1.000000 1 0.765 0.5 0.700000 2 0.800 0.7 0.180000
Both mean and min-max normalization techniques ensure that each column's values fall within the range [0, 1], facilitating data comparison and analysis. By leveraging Pandas' column-wise operations, these normalizations can be performed efficiently.
The above is the detailed content of How to Normalize Columns of a Dataframe in Python?. For more information, please follow other related articles on the PHP Chinese website!
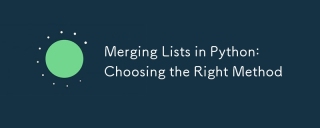
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
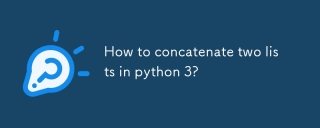
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
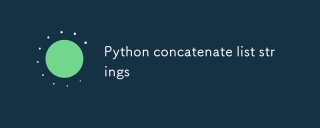
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
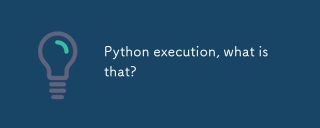
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
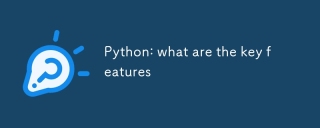
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
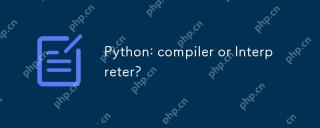
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
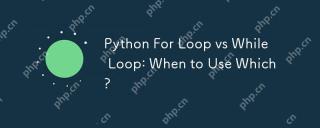
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
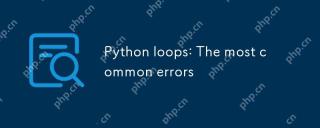
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
