This article introduces the basic concepts and structures of Java programming. It begins with an introduction to variables and data types, then discusses operators and expressions, and control flow processes. Second, it explains methods and classes, then introduces input and output operations. Finally, the article demonstrates the application of these concepts through a practical example of a salary calculator.
Think like a programmer: Master the basics of Java
1. Variables and data types
Java uses variables to store information. Each variable has a specific data type that indicates the type of value it can store. For example:
int age = 30; // 存储整数 String name = "John"; // 存储字符串 double balance = 100.50; // 存储小数
2. Operators and expressions The
operator is used to perform operations on variables. Expressions combine operators with variables or values:
int sum = age + 10; // 加法操作符 boolean isAdult = age >= 18; // 关系操作符 double discount = balance * 0.1; // 乘法操作符
3. Control flow
Control flow statements are used to control the execution flow of a program:
if (isAdult) { System.out.println("You are an adult."); } else { System.out.println("You are not an adult."); } for (int i = 0; i < 5; i++) { System.out.println(i); }
4. Methods and Classes
Methods are reusable blocks of code that perform specific tasks. Classes are blueprints for objects. They define the state and behavior of the object:
class Person { private int age; private String name; public Person(int age, String name) { this.age = age; this.name = name; } public int getAge() { return age; } public String getName() { return name; } }
5. Input and Output
Java provides functions for reading input from the console and writing to it Output method:
Scanner input = new Scanner(System.in); // 读取输入 int inputAge = input.nextInt(); System.out.println("Your age is: " + inputAge); // 写入输出
Practical case: Salary Calculator
import java.util.Scanner; public class SalaryCalculator { public static void main(String[] args) { Scanner input = new Scanner(System.in); // 输入小时数和时薪 System.out.println("Enter the number of hours worked:"); int hoursWorked = input.nextInt(); System.out.println("Enter the hourly pay rate:"); double hourlyRate = input.nextDouble(); // 计算工资 double grossSalary = hoursWorked * hourlyRate; // 输出工资 System.out.println("Your gross salary is: " + grossSalary); } }
The above is the detailed content of Think Like a Programmer: Learning the Fundamentals of Java. For more information, please follow other related articles on the PHP Chinese website!
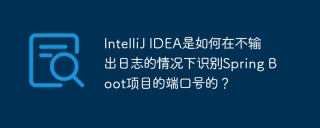
Start Spring using IntelliJIDEAUltimate version...
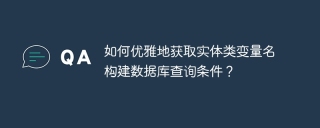
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
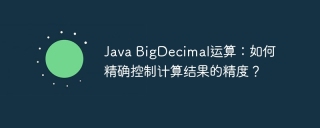
Java...
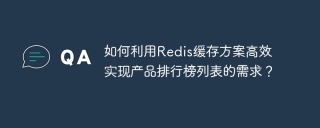
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
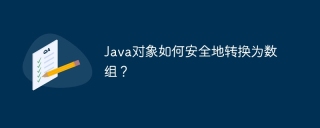
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
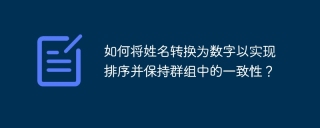
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
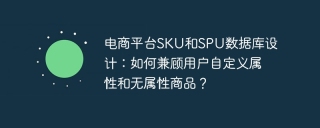
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
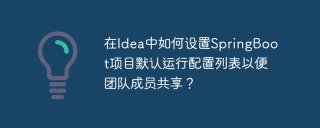
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
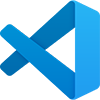
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
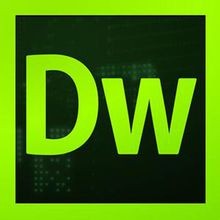
Dreamweaver CS6
Visual web development tools